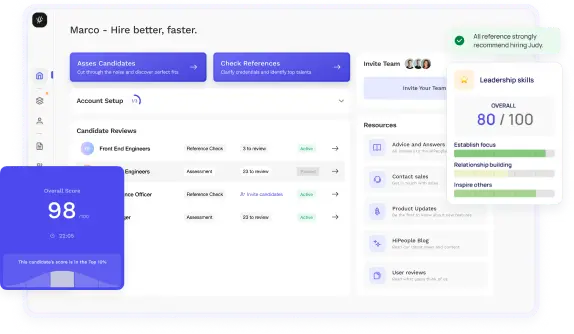
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Ever wondered what it takes to ace a C programming interview? With its deep roots in system-level programming and high-performance computing, C remains a fundamental language in the tech industry. As a candidate, understanding the nuances of C programming and preparing for its unique challenges can significantly impact your success in interviews. For employers, crafting effective interview questions and evaluating candidates’ proficiency requires a well-rounded approach. This comprehensive guide delves into essential C programming interview topics, offering insights and strategies to help both candidates and employers navigate the interview process with confidence. Whether you're preparing to showcase your skills or assessing potential hires, mastering these concepts will be key to achieving your goals.
The C programming language, developed in the early 1970s by Dennis Ritchie at Bell Labs, has significantly influenced the development of modern computing. Despite being several decades old, C remains a cornerstone of software development due to its efficiency, portability, and versatility. This overview explores the continued relevance of C and its impact on various domains within the technology landscape.
C’s design philosophy, which emphasizes efficiency and control over system resources, has established it as a fundamental language in the history of computing. Initially created as an implementation language for the UNIX operating system, C quickly gained traction for its powerful features and relatively straightforward syntax. Over the years, C has evolved through various standards, including ANSI C (C89/C90), C99, C11, and C18, each introducing enhancements and new features to keep the language relevant.
C is known for its close-to-the-metal programming capabilities, allowing developers to write system-level code with minimal overhead. Key features include:
Despite the rise of higher-level languages, C remains a vital tool in modern software development. Its use spans several domains, from embedded systems to high-performance computing. Here’s a look at its various applications:
C has also significantly influenced many other programming languages. Languages such as C++, C#, and Objective-C extend C’s syntax and features while introducing additional capabilities. Understanding C provides a solid foundation for learning these related languages and helps in grasping core programming concepts.
C continues to be a critical language in modern software development for several reasons:
By maintaining its relevance across diverse applications and industries, C continues to play a crucial role in modern software development, proving its enduring value and impact in the field.
Mastering the core concepts of C programming is crucial for both acing your interview and demonstrating a strong understanding of the language. This section delves into fundamental areas that you need to be well-versed in: core language features and syntax, memory management, and data structures.
To thrive in a C programming interview, you must be fluent in the language’s core features and syntax. This includes understanding how C programs are structured and how different elements of the language interact.
Memory management is a fundamental aspect of C programming, and pointers play a crucial role in how memory is handled. Proficiency in these areas will help you write efficient and error-free code.
malloc
, calloc
, realloc
, and free
for managing dynamic memory. Knowing how to allocate and deallocate memory dynamically is vital for handling data structures and managing resources efficiently. Understanding the implications of memory leaks and how to avoid them is also important.Data structures are the building blocks of efficient algorithms and are essential for problem-solving in interviews. Understanding how to implement and use various data structures will enhance your ability to solve complex problems.
Algorithms are a crucial aspect of programming interviews, and knowing how to implement them efficiently in C is important. This involves not only understanding different algorithms but also how to apply them effectively.
By mastering these essential concepts and practicing their application, you will be well-prepared to handle C programming interviews and demonstrate your proficiency effectively.
How to Answer: Begin by discussing basic memory allocation in C, differentiating between malloc() and calloc(). Mention their syntax, the type of memory they allocate, and any initializations performed by these functions. You can also briefly provide examples.
Sample Answer: "Yes, malloc() and calloc() are both used for dynamic memory allocation in C. The key differences lie in their initialization and syntax. malloc(size) allocates a block of memory of size bytes and leaves the memory uninitialized, meaning it contains garbage values. On the other hand, calloc(num, size) allocates memory for an array of elements, each of size bytes, and initializes the allocated memory to zero. For example, malloc(10*sizeof(int)) would allocate memory for 10 integers without initialization, whereas calloc(10, sizeof(int)) would allocate and initialize it to zero."
What to Look For: Look for a clear understanding of the differences between malloc() and calloc(), their respective syntax, and how they handle memory initialization. The candidate may also provide a practical example to reinforce their understanding.
How to Answer: Discuss the concept of pointers within the context of C programming. Highlight how pointers are used to store memory addresses, how they can be dereferenced, and common operations associated with them. You can also touch on their importance in functions and arrays.
Sample Answer: "Pointers are variables that store the address of another variable. In C, they play a crucial role in dynamic memory allocation, parameter passing, and complex data structures like linked lists. For instance, int *p; declares a pointer to an integer. You can assign it the address of a variable using the address-of operator, like p = &var;, and access the value stored at that address using the dereferencing operator, such as *p. Pointers can also be used to pass large structures to functions without copying them, thus improving performance."
What to Look For: Ensure the candidate demonstrates a thorough understanding of pointers, including their declaration, initialization, operations, and practical applications. Their response should reflect their ability to use pointers for efficient coding practices.
How to Answer: Explain the concept of static variables and their scope and lifetime. Highlight specific scenarios where static variables are advantageous, such as in functions or maintaining state across multiple function calls.
Sample Answer: "Static variables in C retain their value between function calls and have a scope limited to the file, block, or function in which they are declared. For example, a static variable within a function will keep its value between invocations of that function. This is useful for maintaining state without using global variables. In practice, declaring a variable as static int counter; inside a function initializes it only once, and it retains its incremented value across multiple calls to that function."
What to Look For: Look for an understanding of the static variable's scope and lifecycle. A good answer will also show the candidate's ability to decide when to use static variables appropriately, demonstrating their grasp of memory and state management in programs.
How to Answer: Define the volatile keyword and explain its use in preventing optimization by the compiler. Provide scenarios, such as dealing with hardware registers or shared variables in a multi-threaded environment, and explain why volatile is necessary in these contexts.
Sample Answer: "The volatile keyword tells the compiler that a variable's value may change at any time, without any action being taken by the code the compiler finds nearby. This is particularly relevant in embedded systems, where a variable may change due to hardware actions. For instance, volatile int timer; would ensure that the compiler always reads the actual value from memory rather than using a cached value, useful for variables updated by an interrupt routine or hardware register."
What to Look For: Ensure the candidate understands the purpose of the volatile keyword and can identify situations where it is necessary. Look for explanations involving real-world scenarios, like hardware interfacing or concurrent programming.
How to Answer: Outline a step-by-step approach to identifying and fixing segmentation faults, including using debugging tools like gdb, examining core dumps, checking pointer usage, and ensuring proper memory access.
Sample Answer: "To address a segmentation fault, I would first use a debugger like gdb to pinpoint exactly where the fault occurs. Running gdb ./program and then run can provide valuable insight when the fault happens. The next step is to check for common issues such as dereferencing null or uninitialized pointers, accessing memory out of bounds, or faulty dynamic memory allocation. Carefully inspecting code sections that handle pointers and array indexing typically reveals the issue. Ensuring pointers are correctly allocated and deallocated can also prevent segmentation faults."
What to Look For: Look for a methodical approach to debugging, including the use of appropriate tools and techniques. The candidate should understand common causes of segmentation faults and how to diagnose and fix them.
How to Answer: Discuss various optimization techniques, including code profiling, algorithm optimization, memory management, compiler optimizations, and specific coding practices that enhance performance.
Sample Answer: "Optimizing a C program starts with profiling the code to identify bottlenecks using tools like gprof or valgrind. Based on profiling results, I would focus on optimizing critical sections of the code. This may involve choosing more efficient algorithms, minimizing memory access, and using faster data structures. Compiler optimization flags such as -O2 or -O3 can also be helpful. Additionally, I might use inlining for small, frequently called functions, and loop unrolling to reduce overhead. It's essential to measure performance improvements after each change."
What to Look For: Ensure the candidate demonstrates a systematic approach to optimization, including profiling, analysis, and specific techniques. Look for an understanding of both code-level and compiler-level optimizations.
How to Answer: Explain the basic structure of a linked list, including how nodes are created and linked. Discuss insertion, deletion, and traversal operations, providing code snippets to illustrate your points.
Sample Answer: "A linked list in C consists of nodes that contain data and a pointer to the next node. The basic structure of a node can be defined as struct Node { int data; struct Node* next; };. To insert a new node at the beginning, I would create a new node and update its next pointer to the current head of the list, like so:
void insertAtBeginning(struct Node** head, int newData) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = newData;
newNode->next = *head;
*head = newNode;
}
Traversal involves iterating through each node until the end of the list, checking and updating pointers. Deletion requires careful handling of pointers to avoid memory leaks."
What to Look For: Look for a clear understanding of the linked list structure, operations, and pointer manipulation. Code examples can demonstrate the candidate’s practical skills in implementing linked lists.
How to Answer: Describe the fundamental differences between stacks (LIFO) and queues (FIFO). Discuss typical operations such as push, pop, enqueue, and dequeue. Provide example code snippets to illustrate the implementation.
Sample Answer: "Stacks follow Last In, First Out (LIFO) principles, while queues follow First In, First Out (FIFO). In C, a stack can be implemented using an array or linked list. For a simple array-based stack:
#define MAX 100
int top = -1;
int stack[MAX];
void push(int value) {
if (top == MAX - 1) printf("Stack overflow\n");
else stack[++top] = value;
}
int pop() {
if (top == -1) printf("Stack underflow\n");
else return stack[top--];
}
Queues can also be implemented using arrays or linked lists. For an array-based queue:
int front = -1, rear = -1;
void enqueue(int value) {
if (rear == MAX - 1) printf("Queue full\n");
else if (front == -1) front = rear = 0;
else queue[++rear] = value;
}
int dequeue() {
if (front == -1 || front > rear) printf("Queue empty\n");
else return queue[front++];
}
Handling full and empty conditions is essential for both structures."
What to Look For: Ensure the candidate can articulate the differences between stacks and queues. Look for correct implementations and understandings of basic operations and boundary conditions for both data structures.
How to Answer: Explain the necessity of synchronization to prevent race conditions. Discuss synchronization mechanisms in C, such as mutexes, semaphores, and condition variables, providing example usage.
Sample Answer: "Synchronization is crucial in multi-threaded programs to avoid race conditions, where multiple threads access shared resources concurrently. In C, pthreads provide synchronization primitives. For instance, using a mutex:
pthread_mutex_t lock;
void *threadFunction(void *arg) {
pthread_mutex_lock(&lock);
// Critical section
pthread_mutex_unlock(&lock);
return NULL;
}
int main() {
pthread_t thread1, thread2;
pthread_mutex_init(&lock, NULL);
pthread_create(&thread1, NULL, threadFunction, NULL);
pthread_create(&thread2, NULL, threadFunction, NULL);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
pthread_mutex_destroy(&lock);
return 0;
}
Mutexes lock critical sections to ensure only one thread accesses shared data at a time. Semaphores and condition variables offer more complex synchronization."
What to Look For: Look for an understanding of race conditions and why synchronization is needed. Ensure the candidate knows how and when to use different synchronization mechanisms, demonstrating this with practical examples.
How to Answer: Define deadlocks and discuss various strategies to prevent them. These can include lock ordering, lock timeout, deadlock detection, and resource allocation strategies.
Sample Answer: "A deadlock occurs when two or more threads are waiting indefinitely for resources held by each other. To prevent deadlocks, I follow several strategies:
What to Look For: Ensure the candidate understands deadlocks, their causes, and practical strategies to prevent them. Look for a clear explanation and reasoning behind their approach, demonstrating practical knowledge with examples.
How to Answer: Discuss key principles of writing maintainable code, such as readability, modularity, documentation, and adherence to coding standards. Provide examples of how you implement these practices in your programming.
Sample Answer: "Writing maintainable C code involves several best practices. First, I emphasize readability by using meaningful variable names and consistent indentation. Breaking down the code into modular functions promotes reusability and easier debugging. For instance, instead of writing one large function, I divide it into smaller, single-responsibility functions. Comments and documentation are crucial for explaining complex logic. Adhering to coding standards, such as those defined by MISRA C or custom project guidelines, ensures consistency across the codebase. Using static code analysis tools helps catch common issues early, enhancing maintainability."
What to Look For: Look for an understanding of best practices in software development. Ensure the candidate values readability, modularity, documentation, and adherence to coding standards and can articulate how these are applied in their work.
How to Answer: Explain your approach to error handling using return codes, errno, and custom error messages. Discuss techniques and tools you use for debugging, such as gdb, valgrind, assertions, and logging.
Sample Answer: "In C, I handle errors using return codes and the errno variable for standard library functions. For example, I design functions to return a success/failure status and use errno to convey specific error details. Here's a snippet:
FILE *file = fopen("file.txt", "r");
if (file == NULL) {
perror("Error opening file");
return -1;
}
For debugging, I rely on gdb to step through code and inspect variables interactively. Valgrind helps detect memory leaks and other issues. Assertions (assert()) are valuable for catching bugs during development. Logging critical events helps trace errors in production environments. For instance, I use syslog() or custom logging mechanisms to record error states."
What to Look For: Ensure the candidate understands various error-handling techniques and their importance. Look for familiarity with debugging tools and strategies, reflecting their practical experience in identifying and fixing issues.
How to Answer: Detail a structured approach to debugging, including reproducing the issue, isolating the problem, using debugging tools, checking code revisions, adding diagnostic messages, and systematically verifying each component.
Sample Answer: "When debugging a complex C program, I start by reproducing the issue consistently. This helps isolate the conditions under which the problem occurs. I break down the program into smaller components to identify the faulty section. Using tools like gdb, I set breakpoints and step through the code to observe variable values and control flow. Reviewing recent code revisions often sheds light on new bugs. Adding diagnostic messages or using logging libraries helps track the program's state. If necessary, I use valgrind to check for memory issues. I systematically verify each module, ensuring all assumptions hold true."
What to Look For: Look for a systematic approach to debugging, including practical use of tools and methods. The candidate should demonstrate critical thinking and attention to detail, along with a logical progression in their debugging strategy.
How to Answer: Discuss various optimization techniques, including code profiling, algorithm optimization, memory management, compiler optimizations, and specific coding practices that enhance performance.
Sample Answer: "Optimizing a C program starts with profiling the code to identify bottlenecks using tools like gprof or valgrind. Based on profiling results, I would focus on optimizing critical sections of the code. This may involve choosing more efficient algorithms, minimizing memory access, and using faster data structures. Compiler optimization flags such as -O2 or -O3 can also be helpful. Additionally, I might use inlining for small, frequently called functions, and loop unrolling to reduce overhead. It's essential to measure performance improvements after each change."
What to Look For: Ensure the candidate demonstrates a systematic approach to optimization, including profiling, analysis, and specific techniques. Look for an understanding of both code-level and compiler-level optimizations.
How to Answer: Discuss the functions used for dynamic memory allocation in C (malloc(), calloc(), realloc(), free()), highlighting their purposes and typical usage. Provide examples to illustrate memory allocation, reallocation, and deallocation.
Sample Answer: "Dynamic memory allocation in C involves allocating memory at runtime using the functions malloc(), calloc(), and realloc(). malloc(size) allocates a memory block of size bytes, while calloc(num, size) allocates memory for an array of num elements, initializing them to zero. realloc(ptr, size) resizes the memory block pointed to by ptr to size bytes. Allocated memory should be freed using free(ptr) to avoid memory leaks. For example:
int *arr = malloc(10 * sizeof(int));
if (!arr) { printf("Memory allocation failed\n"); exit(1); }
arr = realloc(arr, 20 * sizeof(int));
if (!arr) { printf("Memory reallocation failed\n"); exit(1); }
free(arr);
What to Look For: Ensure the candidate understands the roles of different memory allocation functions and when to use them. Look for an awareness of memory management practices, including the importance of freeing allocated memory to avoid leaks.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Mastering advanced topics in C programming can significantly enhance your ability to solve complex problems and optimize your code. These areas include dynamic memory allocation, multi-threading, system-level programming, and low-level optimization techniques. Delving into these subjects will provide a deeper understanding of how C interacts with system resources and how to write high-performance applications.
Dynamic memory allocation allows for the flexible management of memory, which is crucial when dealing with varying sizes of data at runtime. Understanding how to allocate, manage, and free memory dynamically is essential for building efficient and robust C programs.
malloc
, calloc
, realloc
, and free
. malloc
allocates a specified number of bytes and returns a pointer to the allocated memory. calloc
allocates memory for an array of elements and initializes them to zero. realloc
resizes a previously allocated memory block, and free
deallocates memory that was previously allocated. Proper use of these functions is crucial for avoiding memory leaks and ensuring efficient memory use.Multi-threading and concurrency allow programs to perform multiple tasks simultaneously, which can lead to significant performance improvements. However, managing concurrent operations introduces complexity, including issues related to synchronization and data consistency.
pthread_create
, pthread_join
, and pthread_exit
are used to create, manage, and terminate threads. Understanding how to use these functions is essential for implementing concurrent operations in C.System-level programming involves writing software that interacts closely with the operating system and hardware. POSIX threads (pthreads) are a key component of system-level programming in C, providing a way to perform multi-threaded operations across different platforms.
open
, read
, write
, and close
, is essential for writing system-level code that interacts with the OS.fcntl
and ioctl
provide additional control over file descriptors and device operations.pthread_create
, synchronizing threads with mutexes and condition variables, and managing thread attributes. Understanding how to use pthreads effectively can help you build robust, multi-threaded applications that perform well under various conditions.Low-level programming involves working closely with hardware and system architecture to achieve fine-tuned performance and efficiency. Optimization techniques focus on improving the execution speed and reducing the resource usage of your programs.
By diving into these advanced topics and techniques, you’ll be equipped to handle complex programming challenges, optimize performance, and write efficient, high-quality C code.
Preparing thoroughly for a C programming interview involves more than just reviewing technical concepts. It requires a strategic approach to ensure you cover all necessary areas and approach the interview with confidence. Here’s a comprehensive list of best practices to help you excel:
malloc
, calloc
, realloc
, and free
to handle dynamic memory effectively. Be aware of common pitfalls such as memory leaks and segmentation faults.By following these best practices, you will enhance your preparation, improve your performance in C programming interviews, and increase your chances of securing the job you want.
Understanding the interview process and the criteria for evaluating candidates can help both interviewees and interviewers approach the process more effectively. This section delves into the common formats and structures of interviews, key skills and attributes that employers seek, how to assess technical competence and problem-solving skills, and the importance of evaluating cultural fit and soft skills.
C programming interviews typically follow a structured format designed to assess both technical skills and problem-solving abilities. Familiarizing yourself with these formats can help you prepare more effectively.
Employers look for a combination of technical expertise and personal qualities when evaluating candidates. Understanding these desired skills and attributes can help you tailor your preparation and presentation.
Evaluating a candidate’s technical competence and problem-solving abilities involves assessing both their knowledge and their approach to solving problems. Here are key aspects to consider:
Cultural fit and soft skills are crucial for long-term success in a role. Evaluating these aspects helps ensure that candidates align with the company’s values and can work effectively within the team.
By understanding the interview process, knowing what skills and attributes to look for, and assessing both technical competence and cultural fit, you can approach interviews more effectively and make informed decisions about potential hires or prepare yourself to stand out as a candidate.
Whether you're preparing for a C programming interview as a candidate or conducting one as an employer, adopting the right strategies can greatly enhance your effectiveness and outcomes. Below are essential tips to help both candidates and employers navigate the interview process more smoothly and achieve better results.
By incorporating these tips, candidates can better prepare for their interviews and present themselves effectively, while employers can create a more effective and fair interview process that accurately evaluates candidates' skills and fit for the role.
Understanding and preparing for C programming interview questions involves more than just reviewing syntax and coding problems. It requires a thorough grasp of core concepts, from memory management and data structures to advanced topics like multi-threading and system-level programming. By familiarizing yourself with these areas and practicing a wide range of problems, you can approach interviews with greater confidence and demonstrate your ability to handle real-world challenges. For candidates, the key is to focus on both technical and problem-solving skills, while also being prepared to discuss your approach and reasoning. Employers, on the other hand, should design interview processes that effectively evaluate these skills, ensuring a fair and comprehensive assessment of each candidate’s capabilities.
Equally important is the ability to communicate clearly and effectively during the interview. Both candidates and employers benefit from a structured and respectful interview process that allows for a thorough evaluation of technical skills and cultural fit. For candidates, this means articulating your thought process, discussing problem-solving strategies, and showcasing your adaptability. Employers should focus on creating a positive interview experience, providing clear instructions, and assessing both technical expertise and soft skills. By adhering to these practices, you can enhance the overall effectiveness of the interview process and ensure a successful match between candidates and roles.