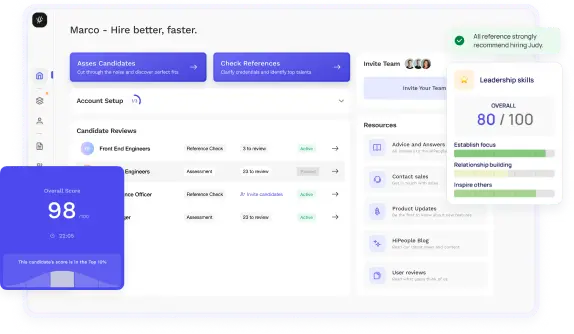
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Ever wondered how to navigate through Git interview questions with confidence and precision? Mastering Git—a cornerstone of modern software development—is crucial for both employers seeking skilled candidates and developers aiming to showcase their expertise. This guide dives deep into the essential concepts, practical skills, and strategies necessary to excel in Git interviews. Whether you're preparing to assess candidates' Git proficiency or aiming to prove your capabilities, this guide equips you with the knowledge and insights needed to succeed in today's competitive tech landscape.
Git interviews assess candidates' proficiency in using Git—a distributed version control system essential for managing codebases in software development. These interviews aim to evaluate candidates' understanding of Git concepts, practical experience, and ability to apply Git in real-world scenarios.
Git interviews serve distinct purposes for both employers and candidates, focusing on different aspects that contribute to successful software development collaborations:
Understanding the significance of Git in software development and the specific goals of Git interviews helps both employers and candidates prepare effectively for the evaluation process. Employers seek candidates who can contribute effectively to team projects through proficient Git usage, while candidates aim to demonstrate their capabilities and stand out as valuable assets in collaborative software development environments.
Git serves as the backbone of version control in software development, enabling teams to collaborate seamlessly and manage code changes efficiently. Here’s a deep dive into the fundamental aspects of Git that every developer should understand.
Git is a distributed version control system designed by Linus Torvalds, initially for managing the Linux kernel development. It tracks changes in source code during software development, facilitating collaboration among multiple developers working on the same project. Unlike centralized version control systems (e.g., SVN), Git allows each developer to have a local copy of the entire repository, complete with its history of changes.
A Git repository (or repo) is a data structure that stores metadata and object database for the project. It contains:
Commits represent snapshots of the repository at different points in time. Each commit contains:
Branches in Git are independent lines of development that allow developers to work on features or fixes without affecting the main codebase. Key concepts include:
git branch
).git checkout
).git merge
).Merging integrates changes from one branch into another, ensuring that multiple developers' work remains synchronized. Git supports different types of merges, such as:
Mastering Git commands is essential for navigating through version control tasks efficiently. Here are some fundamental commands and operations:
git add .
for all changes).git commit -m "Commit message"
).git push origin master
to push changes to the master branch).Understanding these concepts and commands lays a strong foundation for effectively using Git in collaborative software development projects. Each aspect—repositories, commits, branches, and merges—plays a vital role in maintaining version history, facilitating teamwork, and ensuring code stability.
How to Answer: Explain that Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. Highlight its key features, such as branching, merging, and repository cloning.
Sample Answer:
"Git is a distributed version control system that helps track changes in source code during software development. It allows multiple developers to work on a project simultaneously without overwriting each other's changes. Key features include branching, which allows for isolated development, and merging, which integrates changes from different branches. Git's efficiency and flexibility make it a preferred tool for developers worldwide."
What to Look For: Look for a clear understanding of Git's purpose and features. Candidates should demonstrate knowledge of how Git benefits software development processes, particularly in collaborative environments.
How to Answer: Clarify that Git is the version control system, while GitHub is a platform for hosting Git repositories, providing additional features like collaboration tools, issue tracking, and web-based interfaces.
Sample Answer:
"Git is a version control system that manages changes to source code over time, allowing multiple developers to work on a project simultaneously. GitHub, on the other hand, is a cloud-based hosting service for Git repositories. It provides additional features such as pull requests, issue tracking, and project management tools. Essentially, Git is the tool, and GitHub is a service that leverages Git to facilitate collaborative development."
What to Look For: Ensure the candidate distinguishes between the functionalities of Git and GitHub and understands how they complement each other in software development.
How to Answer: Describe the command git init and explain its usage in creating a new repository.
Sample Answer:
"To initialize a new Git repository, you use the command git init. This command creates a new subdirectory named .git that contains all the necessary metadata and version control information for the repository. For example, running git init my-project will set up a new Git repository in the my-project directory."
What to Look For: Candidates should be familiar with the git init command and understand its purpose. They should also be able to describe the structure of a Git repository.
How to Answer: Explain the process of staging with git add and committing with git commit, including options for commit messages.
Sample Answer:
"To stage changes in Git, you use the git add command followed by the file names you want to stage. For example, git add file1.txt file2.txt stages file1.txt and file2.txt for the next commit. Once the changes are staged, you use git commit -m 'Your commit message' to commit them. This command records the changes along with a descriptive message. For example, git commit -m 'Fixed the bug in file1 and updated file2'."
What to Look For: Look for an understanding of the staging and committing process. Candidates should know the purpose of staging changes and the importance of meaningful commit messages.
How to Answer: Define what a branch is and describe the command git branch to create a new one.
Sample Answer:
"A branch in Git is a separate line of development that allows you to work on new features or bug fixes without affecting the main codebase. To create a new branch, you use the git branch command followed by the branch name. For example, git branch feature-x creates a new branch named feature-x. You can switch to this branch using git checkout feature-x."
What to Look For: Ensure the candidate understands the concept of branches and the commands to create and switch between them. They should also appreciate the role of branches in parallel development.
How to Answer: Describe the process of merging with the git merge command and discuss potential conflicts.
Sample Answer:
"To merge a branch into the main branch, you first switch to the main branch using git checkout main. Then, you use the git merge command followed by the branch name you want to merge. For example, git merge feature-x merges the feature-x branch into the main branch. If there are conflicts, Git will notify you, and you will need to resolve them manually before completing the merge."
What to Look For: Candidates should demonstrate knowledge of the merging process and conflict resolution. Look for an understanding of how merging integrates different development lines and handles conflicts.
How to Answer: Explain the git clone command and its options.
Sample Answer:
"To clone a remote repository, you use the git clone command followed by the repository URL. For example, git clone https://github.com/user/repo.git creates a local copy of the repository. The command also supports options like specifying a directory name with git clone https://github.com/user/repo.git my-directory."
What to Look For: Ensure the candidate knows how to use the git clone command and understands its purpose. They should be able to explain how cloning sets up a local repository linked to the remote.
How to Answer: Describe the git push command and its usage for updating remote branches.
Sample Answer:
"To push changes to a remote repository, you use the git push command followed by the remote name and branch name. For example, git push origin main pushes the changes from your local main branch to the main branch on the remote named origin. If it's the first time you're pushing to this branch, you may need to set the upstream with git push --set-upstream origin main."
What to Look For: Look for understanding of the git push command and its role in synchronizing local changes with a remote repository. Candidates should also be aware of how to set the upstream branch.
How to Answer: Explain the concept of rebasing and how it differs from merging, including scenarios where each is appropriate.
Sample Answer:
"Git rebase is a way to integrate changes from one branch into another by moving or combining a sequence of commits. Unlike merging, which creates a new commit to combine branches, rebasing re-applies commits on top of another base commit. This results in a linear project history. You use git rebase followed by the branch name, for example, git rebase main. Rebasing is useful for maintaining a clean project history but should be used carefully to avoid rewriting shared history."
What to Look For: Ensure the candidate understands the differences between rebasing and merging, including the implications for project history. They should know when to use each approach appropriately.
How to Answer: Describe the process of identifying, resolving, and completing merge conflicts.
Sample Answer:
"When a conflict occurs, Git marks the conflicted areas in the affected files. To resolve conflicts, you need to open the files, find the conflict markers, and manually edit the code to resolve the issues. Once the conflicts are resolved, you stage the changes with git add and complete the merge with git commit. Tools like git status can help identify conflicted files, and graphical tools or IDEs often provide conflict resolution interfaces."
What to Look For: Candidates should demonstrate a clear understanding of conflict resolution, including how to identify and resolve conflicts manually. They should also be aware of tools that can assist in this process.
How to Answer: Explain the general process of how Git is used in a development workflow, including steps like branching, committing, pushing, and merging.
Sample Answer:
"The Git workflow involves a series of steps that developers follow to manage their code changes. Typically, it starts with creating a branch for a new feature or bug fix. Changes are made and committed locally, and then pushed to a remote repository. Other developers can review and merge these changes into the main branch. This workflow ensures a structured and collaborative development process, minimizing conflicts and maintaining code quality."
What to Look For: Look for an understanding of the structured approach in using Git, emphasizing the benefits of organized code management and collaboration.
How to Answer: Discuss strategies like communication, branching, frequent commits, and resolving conflicts.
Sample Answer:
"When multiple developers need to work on the same file, effective communication and coordination are crucial. Developers should frequently commit and push their changes to minimize conflicts. Branching can also help by allowing isolated development. In case of conflicts, resolving them promptly and discussing the changes with the team can ensure smooth integration."
What to Look For: Ensure the candidate understands collaborative strategies and the importance of communication. They should also know practical techniques for minimizing and resolving conflicts.
How to Answer: Explain the git config command and its options for setting username and email.
Sample Answer:
"To configure your Git username and email, you use the git config command. For example, git config --global user.name 'Your Name' sets the username, and git config --global user.email 'your.email@example.com' sets the email address. Using the --global option applies these settings globally for all repositories."
What to Look For: Look for an understanding of the git config command and its role in setting user-specific configurations.
How to Answer: Describe the git config --list command and its usage.
Sample Answer:
"To view the current Git configuration settings, you use the git config --list command. This command displays a list of all the settings Git is using, including user information, aliases, and other configurations. It's a useful way to verify your setup and make sure everything is configured correctly."
What to Look For: Candidates should know how to check and verify their Git configurations and understand the significance of these settings.
How to Answer: Explain the concept of Git aliases and how to set them using the git config command.
Sample Answer:
"A Git alias is a shortcut that allows you to use shorter commands for frequently used Git operations. To create an alias, you use the git config command. For example, git config --global alias.co checkout creates an alias co for the checkout command. This way, you can use git co instead of git checkout, saving time and keystrokes."
What to Look For: Ensure the candidate understands the purpose of aliases and knows how to create and use them effectively.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Preparing for a Git interview involves more than just memorizing commands; it requires a deep understanding of Git concepts, practical experience, and the ability to articulate your knowledge effectively. Here’s how you can ensure you’re well-prepared to ace your Git interview.
Mastering Git goes beyond knowing basic commands; it involves understanding Git's architecture, workflows, and best practices:
git init
, git clone
, git add
, git commit
, git push
, git pull
, and git merge
.To build and enhance your Git skills, leverage a variety of learning resources tailored to different learning styles and preferences:
Hands-on experience is invaluable when preparing for a Git interview. Engage in practical exercises and projects to apply theoretical knowledge and develop problem-solving skills:
By combining theoretical knowledge with practical experience and leveraging diverse learning resources, you'll build confidence in your Git proficiency and be well-prepared to showcase your skills in any Git interview scenario.
Preparing to demonstrate your Git skills in an interview involves more than just technical prowess—it's about showcasing your ability to apply Git effectively in real-world scenarios and adhere to best practices. Here’s how you can impress interviewers with your Git expertise.
Highlighting your practical experience with Git projects is essential to demonstrate your proficiency:
Discussing Git best practices showcases your attention to detail and professionalism in managing code:
Demonstrating your ability to troubleshoot and resolve Git-related challenges showcases your problem-solving skills:
By demonstrating your practical experience with Git projects, adherence to best practices, and ability to tackle challenges effectively, you’ll showcase your readiness to contribute to development teams and handle complex projects with confidence. Prepare specific examples that highlight your strengths and align with the job requirements to make a lasting impression during your Git interview.
As an employer, evaluating Git skills in candidates goes beyond assessing their knowledge of commands. It involves understanding their ability to use Git effectively in team environments, adhere to best practices, and troubleshoot common challenges. Here’s how you can effectively evaluate Git proficiency during interviews.
When evaluating Git skills in candidates, consider the following key criteria:
To accurately assess Git proficiency, consider incorporating practical assessments or tests into your interview process:
In addition to technical assessments, use behavioral interview questions to evaluate candidates' Git knowledge and problem-solving approach:
By integrating technical assessments, practical exercises, and behavioral interview questions, you can comprehensively evaluate candidates' Git skills. Look for individuals who not only demonstrate technical proficiency but also exhibit a deep understanding of Git's role in collaborative software development and a commitment to best practices.
Mastering Git for interviews involves more than just memorizing commands—it's about understanding its core principles and applying them effectively. Employers look for candidates who can demonstrate not only technical proficiency but also a deep understanding of Git's role in collaborative software development. By emphasizing practical experience, adherence to best practices, and problem-solving skills in Git-related scenarios, candidates can stand out and showcase their readiness to contribute meaningfully to development teams.
For employers, the ability to assess Git skills accurately ensures that candidates can handle version control effectively, maintain code integrity, and collaborate seamlessly within teams. By incorporating practical assessments, evaluating adherence to Git best practices, and probing candidates' problem-solving abilities through behavioral questions, employers can identify candidates who not only excel in technical aspects but also align with the organization's development goals. Ultimately, mastering Git interviews positions both employers and candidates for successful collaborations and impactful contributions in the dynamic world of software development.