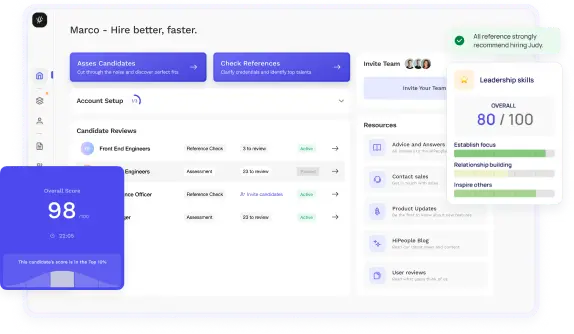
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
How can you stand out in a Golang interview and demonstrate that you’re the right fit for the role? With Go becoming increasingly popular for its performance and simplicity, mastering the intricacies of this language is crucial for both employers and candidates. This guide dives deep into Golang interview questions, providing a thorough overview of essential topics, role-specific focuses, and practical tips for both sides of the table. Whether you're preparing to face challenging technical questions or looking to refine your interviewing process, you'll find valuable insights and actionable advice to navigate Golang interviews effectively.
Golang, commonly known as Go, is a statically typed, compiled programming language designed by Google. It was created to address the shortcomings of existing languages in terms of performance, efficiency, and ease of use. Here’s a comprehensive look at Go, highlighting its features, design principles, and key benefits.
gofmt
automatically format code according to Go’s conventions. This consistency improves collaboration and code quality.Golang has established itself as a significant player in the software development industry, and its importance continues to grow. Here’s why Golang is highly valued:
Golang’s unique features and benefits have cemented its place as a valuable tool in the software development industry, driving its adoption across a diverse range of applications and environments.
How to Answer: Begin by providing a straightforward definition of Golang (Go) and then outline some of its key features such as simplicity, concurrency support, strong static typing, and garbage collection. Mention its suitability for building scalable and high-performance applications, and touch upon its community and ecosystem.
Sample Answer: "Golang, commonly referred to as Go, is an open-source programming language developed by Google. Its main features include a simple and clean syntax that allows developers to write code quickly, powerful concurrency primitives such as goroutines, built-in support for garbage collection, and a strong standard library. Go is designed for building efficient, reliable software, particularly in networked and cloud-based environments, making it ideal for scalable applications."
What to Look For: Look for candidates who clearly understand the language, can articulate its features accurately, and demonstrate familiarity with use cases and applications where Golang excels.
How to Answer: Explain the concept of concurrency as it relates to multi-threading and how Go implements this through goroutines. Highlight the lightweight nature of goroutines and how they are managed by the Go runtime, as well as the concept of channels for communication between goroutines.
Sample Answer: "Golang handles concurrency using goroutines, which are lightweight threads managed by the Go runtime. Unlike traditional threads, goroutines have a smaller memory footprint and are more efficient to create. This allows developers to launch thousands of goroutines without significant performance degradation. Communication between goroutines is typically done through channels, which enable safe data exchange and synchronization, exemplifying Go's implementation of the CSP (Communicating Sequential Processes) model."
What to Look For: Candidates should demonstrate a good understanding of concurrency concepts, the advantages of using goroutines over traditional threading models, and how channels facilitate safe communication. Watch for signs of confusion about alternative concurrency models or poor explanation of goroutines' efficiency.
How to Answer: Discuss the purpose of Go modules for dependency management and explain the steps to create a module, including the go.mod file and how to install dependencies. Mention best practices like versioning and module updating.
Sample Answer: "To create a Go module, you first create a directory for your project and then initialize it with the command go mod init <module_name>. This creates a go.mod file, which tracks the module's dependencies. You can add dependencies using go get <dependency>, which updates your go.mod file automatically. It’s important to manage versions properly by specifying v0.1.0 or later versions, helping maintain stability in larger projects. When making updates, I recommend using go mod tidy to clean up any dependencies that are no longer needed."
What to Look For: Assess whether the candidate has hands-on experience with Go modules and is familiar with best practices in dependency management. A strong candidate should demonstrate understanding not only of the technical steps but also of the implications of versioning and dependency management.
How to Answer: Highlight that error handling in Go is typically done using multiple return values, with the second return value often being of type error. Explain the convention of checking for errors immediately after a function call and how this differs from exceptions in other languages.
Sample Answer: "In Golang, error handling is performed using multiple return values. Functions that can encounter errors usually return a result and an error as the second return value, like so: result, err := someFunction(). It’s a common convention to check if err is not nil immediately after the function call, which allows for direct and clear error handling. This approach emphasizes the importance of handling errors explicitly rather than relying on exceptions, providing developers with more control over flow and functionality."
What to Look For: Candidates should display knowledge of Go's unique error handling pattern and demonstrate clarity in explaining its advantages over traditional exception handling. Look for signs of familiarity with handling complex error types or structured error logging practices.
How to Answer: Discuss idiomatic practices like using clear naming conventions, writing small, modular functions, leveraging interfaces, and keeping error handling explicit. Mention how these idioms contribute to code readability and maintainability.
Sample Answer: "Some common Go idioms I adhere to include using clear and descriptive variable names that reflect their purpose, minimizing function sizes to promote single-responsibility principles, and leveraging interfaces for abstraction. I also consistently handle errors right where they occur to avoid complications later in the code execution. These practices not only enhance readability but also underline the Go philosophy of simplicity and clarity, making it easier for team members to understand and work with the code."
What to Look For: Look for candidates who can articulate specific practices and provide examples of how these idioms impact code quality. Be wary of candidates who struggle to define idioms or cannot relate them to their experiences.
How to Answer: Emphasize the importance of testing in Go, mentioning the built-in testing framework and how to write tests using the testing package. Highlight code reviews, use of linters, and continuous integration as additional factors that contribute to maintaining high code quality.
Sample Answer: "To assure code quality in my Go programs, I make use of the built-in testing framework, which allows me to write unit tests that are straightforward to implement using the testing package. I also perform code reviews with my peers, which helps catch issues early and fosters knowledge sharing within the team. Additionally, employing linters like golint and integrating continuous integration tools like Travis CI ensures that code is consistently checked for coding standards and passing tests before it's deployed."
What to Look For: Candidates should demonstrate knowledge of Go's testing ecosystem and discuss various quality assurance techniques. Ensure they provide comprehensive examples of practices that promote quality and how they have implemented these in past projects.
How to Answer: Define interfaces in the context of Golang as a way to achieve polymorphism. Explain how interfaces specify behavior without dictating implementation and how they enable different types to be used interchangeably when they satisfy an interface contract.
Sample Answer: "In Golang, an interface is a contract that specifies a set of methods. Any type that implements these methods satisfies the interface, allowing for polymorphism. This means I can write functions that take an interface as an argument without needing to know about the underlying type, which enhances flexibility and decouples code. For example, if I have an interface Shape with a method Area(), any struct implementing this can be treated as a Shape, allowing different geometric shapes to be managed seamlessly in my programs."
What to Look For: Candidates should exhibit clarity in explaining the role of interfaces in Go and demonstrate an understanding of how they support clean code and design patterns. Evaluate their familiarity with practical scenarios where interfaces have been beneficial.
How to Answer: Describe Go's memory management model, including stack versus heap allocation and the role of the garbage collector in reclaiming memory. Touch on how Go’s garbage collector is designed for concurrent programming and how it impacts performance.
Sample Answer: "Go manages memory using both stack and heap allocation. Variables allocated on the stack are typically used for short-lived data, while long-lived objects are allocated on the heap. Go employs a garbage collector to automatically reclaim memory that is no longer in use, which reduces memory leaks and manual memory management burdens. The garbage collector is concurrent and incrementally runs while the program executes, minimizing pause times and ensuring that applications maintain high performance even under concurrent load scenarios."
What to Look For: Assess whether candidates demonstrate a thorough understanding of Go's memory management principles and garbage collection process. Watch for any misconceptions about memory handling or failure to address performance implications effectively.
How to Answer: Discuss various profiling tools available in Go, such as the built-in pprof package for CPU and memory profiling. Explain how profiling contributes to identifying bottlenecks and optimizing performance in applications.
Sample Answer: "In Go, I primarily use the built-in pprof package for performance profiling. This tool allows me to analyze CPU and memory usage metrics easily. By integrating pprof into my application, I can generate reports that highlight function call stats and memory allocations, making it easier to pinpoint performance bottlenecks. After analyzing the profiles, I can make data-driven decisions to optimize critical sections of my code, ensuring the application runs efficiently in production environments."
What to Look For: Ensure that candidates not only mention profiling tools but also demonstrate substantive understanding of performance analysis processes. Be cautious of candidates who lack familiarity with profiling in Go or cannot explain how this process can lead to improved performance.
How to Answer: Cover key optimization techniques such as using goroutines to handle concurrency, minimizing latency by implementing caching strategies, and using lightweight frameworks. Discuss the importance of load testing to ensure the server can handle expected traffic.
Sample Answer: "To optimize a web server written in Golang, I utilize goroutines extensively to handle multiple concurrent requests efficiently. Additionally, implementing caching strategies, such as using Redis for frequently requested data, can significantly reduce latency. I also keep an eye on optimizing database queries and responses, ensuring they are as efficient as possible. Lastly, I perform load testing using tools like Apache Benchmark or Artillery to simulate traffic and stress-test the server, helping me identify potential bottlenecks or points of failure before they impact users."
What to Look For: Look for candidates who can articulate multi-faceted strategies for optimization, demonstrating both technical knowledge and practical experience. Pay attention to their awareness of testing and analytics as integral parts of the optimization process.
How to Answer: Describe the importance of constructive communication and respect for differing opinions. Discuss strategies for resolving disagreements, such as open discussions, fact-based analysis, and finding common ground.
Sample Answer: "When disagreements arise within a development team, I believe it's crucial to address them openly and respectfully. I usually initiate a conversation to understand the differing viewpoints and encourage an atmosphere where everyone feels heard. I advocate for fact-based analysis, where we can look at data or code implications together, and work towards finding common ground or a compromise that aligns with our project's goals. Ultimately, fostering collaboration and maintaining a focus on the project’s success helps navigate these disagreements effectively."
What to Look For: Candidates should demonstrate excellent interpersonal skills and a strategic approach to resolving conflict. Be wary of responses that signal a tendency to be dismissive of others’ opinions or avoid confrontation altogether.
How to Answer: Emphasize the importance of knowledge sharing for team growth and effectiveness. Discuss methods such as code reviews, pair programming, and conducting workshops or lunch-and-learns to facilitate learning.
Sample Answer: "I have always valued knowledge sharing as a key component of team dynamics. I often conduct code reviews to provide constructive feedback and encourage junior developers to ask questions, which promotes a learning-centric environment. Additionally, I engage in pair programming sessions where we can collaboratively solve problems, allowing juniors to learn from my approaches directly. I also like to organize informal workshops or 'lunch-and-learns' where I can share insights on specific topics, ensuring that everyone’s skill set continues to grow."
What to Look For: Look for candidate who demonstrate a proactive attitude toward mentoring, collaboration, and knowledge-sharing. Strong candidates will illustrate a history of mentorship and the tangible impact it has had on their teams.
How to Answer: Discuss what initially drew you to Go as a language, such as its syntax, performance capabilities, or community. Reflect on how this choice has shaped your professional development and projects you've been involved in since then.
Sample Answer: "I was initially attracted to Golang because of its simplicity and the strong community backing it received. The clean syntax allows for rapid development, while its excellent concurrency features align with the demands of high-performance applications. Since starting to work with Go, I have been involved in developing scalable microservices that leverage its strengths in handling HTTP requests efficiently. This has significantly enhanced my skills and opened opportunities to work on complex projects, and I've found immense satisfaction in leveraging Go's capabilities in real-world applications."
What to Look For: Candidates should convey genuine enthusiasm for Golang and clearly articulate how it fits into their career trajectory. Look for a connection between their experiences and the language's advantages, indicating a thoughtful choice in their development journey.
How to Answer: Discuss career goals that align with Golang, such as deepening expertise in the language, contributing to open-source projects, or taking on leadership roles. Mention how you plan to continue learning and adapting in a dynamic tech landscape.
Sample Answer: "In the next five years, I envision myself deepening my expertise in Golang and pursuing opportunities to mentor more developers while contributing to open-source projects that enrich the Go ecosystem. I aim to take on more leadership roles, helping shape project architectures that utilize Golang's strengths. Additionally, I will continually seek new learning opportunities, such as attending conferences and participating in relevant workshops, to stay updated with the evolving technology trends and best practices in the Go community."
What to Look For: Candidates should show ambition and a proactive approach to their career in Golang development. Strong responses will indicate a balance between personal growth, mentorship opportunities, and alignment with industry developments.
How to Answer: Provide a high-level overview of designing a RESTful API, covering the architecture, choice of frameworks (like Gin or Echo), routing, request handling, and response formatting. Emphasize best practices such as versioning, authentication, and error responses.
Sample Answer: "To design a RESTful API in Go, I would start by defining the resource structure and the endpoints. I often choose frameworks like Gin or Echo for their simplicity and performance. I would set up routing based on the REST principles, ensuring that each endpoint corresponds to a specific HTTP method operation. Handling requests would involve defining service layers to separate business logic, and structured responses would be returned in JSON format. I would also implement versioning in the URL to facilitate future changes, along with authentication mechanisms such as JWT, and set a standard for error responses to aid client-side error handling."
What to Look For: Candidates should demonstrate an understanding of REST principles and experience in building APIs, emphasizing architectural decisions. Look for practical examples that detail their real-world applications and response structures.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Understanding the core skills and knowledge areas for Golang interviews will set you up for success, whether you're preparing for a role or hiring for one. Golang’s unique features and practical applications require a strong grasp of both fundamental and advanced concepts. Let’s dive into these essential areas.
To excel in a Golang interview, mastering the fundamental concepts of the language is crucial. Golang, designed with simplicity and efficiency in mind, has several core features that you need to be familiar with:
errors
package, is vital.Once you’ve mastered the basics, diving into Go’s advanced features and their practical applications will give you an edge. These features are crucial for tackling complex problems and optimizing performance.
reflect
package, allow you to inspect and manipulate objects at runtime. Reflection can be useful for tasks like creating generic functions, implementing serialization, or building libraries that work with a variety of types. However, reflection should be used judiciously due to its impact on performance and code readability. Demonstrating a balanced use of reflection can showcase your ability to handle dynamic scenarios while maintaining code efficiency.go mod init
, go mod tidy
, and go get
, and understand how to handle module versioning and updates.Leveraging libraries and frameworks can significantly enhance your productivity and the functionality of your Go applications. Here are some commonly used libraries and frameworks you should know about:
net/http
for HTTP server and client implementations, encoding/json
for JSON encoding and decoding, and io
and os
for file and I/O operations. Familiarity with these packages is crucial as they form the backbone of many Go applications.Writing efficient and maintainable code is key to delivering high-quality software. Here are some best practices to follow when coding in Go:
gofmt
tool to automatically format your code according to Go’s conventions. This tool helps ensure that your code adheres to standard practices and makes collaboration easier.testing
package to create unit tests and benchmarks. Writing thorough tests helps catch bugs early, facilitates code refactoring, and ensures that your code behaves as expected.pprof
to analyze CPU and memory usage, and make optimizations based on real data rather than assumptions.By mastering these key skills and practices, you'll be well-prepared for any Golang interview and capable of delivering high-quality, efficient code in your role.
As a backend developer, your primary focus is on building and maintaining the server-side of applications. Golang is a powerful language for backend development due to its efficiency and scalability. Here’s a deep dive into what you need to excel in this role.
In backend development, particularly with Golang, several skills are crucial:
database/sql
package provides a generic interface for SQL databases, and libraries like Gorm simplify database interactions with an ORM approach. Skills in writing efficient SQL queries, managing database connections, and performing migrations are important.pprof
, and techniques for optimizing code performance, including caching strategies and efficient data handling, are key.Backend coding challenges test your ability to design and implement solutions that handle complex requirements:
Understanding algorithms and data structures is crucial for solving complex backend problems:
Real-world case studies help illustrate how theoretical knowledge is applied in practical scenarios:
Systems programmers work on the underlying software that interacts closely with the hardware. Golang’s efficiency and low-level capabilities make it a suitable choice for systems programming.
Systems programming involves working with low-level operations and resource management:
os
and syscall
is important.Concurrency and parallelism are key aspects of systems programming, and Go’s concurrency model provides powerful tools for managing them:
sync.Mutex
or sync/atomic
to manage access to shared data and ensure that concurrent operations do not lead to data corruption or inconsistent states.Optimizing performance in systems programming often involves:
pprof
, to analyze CPU and memory usage. Profiling helps identify performance bottlenecks and areas for optimization.testing
package to measure the performance of specific code segments. Benchmarks provide insights into the efficiency of algorithms and data structures.Designing systems requires addressing various challenges:
In a DevOps role, your focus is on automating and streamlining the software development lifecycle. Golang’s efficiency and versatility can significantly enhance your DevOps practices.
Golang’s features make it well-suited for various DevOps tasks:
Building and deploying Go applications involves several steps:
Integrating Go applications into CI/CD pipelines enhances development efficiency:
Effective troubleshooting and debugging are crucial for maintaining a stable and reliable DevOps environment:
By focusing on these role-specific skills and practices, you’ll be well-prepared to excel in backend development, systems programming, or DevOps roles with Golang.
Conducting effective Golang interviews requires a structured approach to ensure you identify candidates who possess both the technical skills and problem-solving abilities necessary for the role. Here’s a comprehensive list of tips to help you conduct successful Golang interviews:
net/http
, encoding/json
, or database/sql
. This will assess their familiarity with essential libraries and their ability to leverage Go’s built-in capabilities.gofmt
and GoDoc.testing
package and debugging with tools like Delve
. Assess their approach to ensuring code quality and handling runtime issues.
Preparing for a Golang interview involves more than just brushing up on your coding skills. Here’s a comprehensive list of tips to help you get ready and make a strong impression:
net/http
for web development, encoding/json
for data handling, and os
for system operations.
Mastering Golang interview questions involves more than just understanding syntax and features; it requires a deep grasp of how Go’s unique attributes can be applied to solve real-world problems. By familiarizing yourself with Go's core concepts, advanced features, and role-specific applications, you position yourself as a well-rounded candidate or a more effective interviewer. For candidates, focusing on practical coding challenges, system design, and problem-solving will help showcase your proficiency and adaptability. On the other hand, for employers, crafting questions that reflect real-world scenarios and assessing not just technical skills but also problem-solving approaches will lead to a more accurate evaluation of a candidate’s capabilities.
Both sides benefit from a structured approach to Golang interviews. Candidates should leverage the provided tips to prepare thoroughly and address common pitfalls, ensuring they can confidently demonstrate their expertise. Employers, meanwhile, should utilize the outlined strategies to conduct thorough and fair interviews that assess not only technical knowledge but also the ability to apply that knowledge effectively. By adhering to these guidelines, you can significantly enhance the quality of Golang interviews, leading to better hiring decisions and more successful outcomes in the rapidly evolving tech landscape.