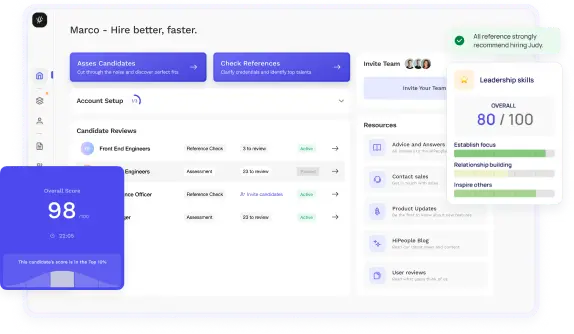
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to ace your Java 8 interview with confidence and expertise? Delve into the guide tailored to both employers seeking top-tier talent and candidates eager to showcase their Java 8 proficiency. From understanding the significance of Java 8 in today's software development landscape to mastering advanced topics like CompletableFuture and the Date and Time API, this guide equips you with the knowledge and practical tips needed to excel in Java 8 interviews. Whether you're brushing up on fundamental concepts or diving into complex Java 8 features, prepare to navigate the interview process with ease and proficiency.
Java 8 marked a significant milestone in the evolution of the Java programming language, introducing several groundbreaking features that have since become integral to modern software development. Let's explore why Java 8 is so significant in the software development landscape:
Java 8 skills are highly valued in the software industry, both by employers seeking skilled developers and by candidates looking to advance their careers. Let's explore the importance of Java 8 skills from both perspectives:
Java 8 brought about a paradigm shift in the Java programming language, introducing several powerful features that have since become integral to modern Java development. Let's take a closer look at these fundamentals.
Java, initially released in 1995 by Sun Microsystems (now owned by Oracle Corporation), quickly rose to prominence as one of the most widely used programming languages in the world. Known for its platform independence, robustness, and scalability, Java has been the backbone of countless enterprise applications, web services, and mobile apps.
Java 8 marked a significant milestone in the evolution of the language, introducing several groundbreaking features that transformed the way developers write code.
The adoption of Java 8 features offers numerous benefits for both developers and organizations:
How to Answer: Lambda expressions in Java 8 provide a concise way to represent anonymous functions. Candidates should explain that lambda expressions facilitate functional programming by enabling the passing of behavior in a more succinct manner. They should discuss the syntax of lambda expressions, including parameter list, arrow token, and body. Emphasize the use of functional interfaces with lambda expressions.
Sample Answer: "A lambda expression in Java 8 is a concise way to represent anonymous functions. It consists of a parameter list, an arrow token (->), and a body. For example, (x, y) -> x + y represents a lambda expression that takes two parameters and returns their sum. Lambda expressions are commonly used with functional interfaces to provide implementations for their abstract methods."
What to Look For: Look for candidates who demonstrate a clear understanding of lambda expressions, including their syntax and usage with functional interfaces. Strong candidates will be able to provide examples and discuss the benefits of lambda expressions in Java programming.
How to Answer: Candidates should explain that default methods in interfaces were introduced in Java 8 to enable adding new methods to interfaces without breaking the classes that implement them. They should discuss how default methods are implemented and how they can be overridden by implementing classes. Emphasize the role of default methods in evolving interfaces without disrupting existing code.
Sample Answer: "Default methods in interfaces are methods with a default implementation that can be directly invoked by implementing classes. They allow interfaces to have method implementations without requiring implementing classes to provide them. Default methods were introduced in Java 8 to enable adding new methods to interfaces without breaking existing code. Implementing classes can choose to override default methods if needed."
What to Look For: Seek candidates who can articulate the purpose and usage of default methods in interfaces. They should understand how default methods facilitate interface evolution and backward compatibility. Look for examples demonstrating the implementation and overriding of default methods.
How to Answer: Candidates should describe the Stream API as a new abstraction introduced in Java 8 to process collections of elements in a functional style. They should explain the key characteristics of streams, such as laziness, immutability, and the ability to perform parallel operations. Emphasize the difference between streams and collections.
Sample Answer: "The Stream API in Java 8 provides a functional approach to process collections of elements. Streams represent a sequence of elements that support various operations like filtering, mapping, and reducing. Streams are characterized by laziness, meaning operations are only executed when a terminal operation is invoked. They are also immutable and can facilitate parallel execution of operations, potentially improving performance."
What to Look For: Look for candidates who can clearly explain the purpose and features of the Stream API. They should understand the differences between streams and collections and be able to discuss the benefits of using streams for processing data. Strong candidates will provide examples demonstrating stream operations.
How to Answer: Candidates should explain that intermediate operations in the Stream API transform or filter the elements of a stream and return a new stream. They should discuss common intermediate operations like map, filter, and sorted. Terminal operations, on the other hand, produce a result or side effect and terminate the stream. Candidates should provide examples of both types of operations.
Sample Answer: "In the Stream API, intermediate operations transform or filter the elements of a stream and return a new stream without affecting the original. Examples of intermediate operations include map, filter, and sorted. Terminal operations, on the other hand, consume the elements of a stream and produce a result or side effect. Common terminal operations include forEach, collect, and reduce."
What to Look For: Seek candidates who demonstrate a solid understanding of intermediate and terminal operations in the Stream API. They should be able to differentiate between the two types of operations and provide examples of each. Look for candidates who can explain how these operations work together in stream processing pipelines.
How to Answer: Candidates should define a functional interface as an interface with exactly one abstract method. They should discuss the @FunctionalInterface annotation and its role in indicating that an interface is intended to be used as a functional interface. Candidates should provide examples of built-in functional interfaces in Java 8.
Sample Answer: "A functional interface in Java is an interface that contains exactly one abstract method. Functional interfaces can have any number of default or static methods, but they must have only one abstract method to qualify as functional. The @FunctionalInterface annotation is used to indicate that an interface is intended to be used as a functional interface. Examples of functional interfaces in Java 8 include Runnable, Consumer, and Predicate."
What to Look For: Look for candidates who can accurately define a functional interface and explain its characteristics. They should understand the purpose of functional interfaces in enabling lambda expressions and functional programming in Java. Strong candidates will provide examples and discuss the role of the @FunctionalInterface annotation.
How to Answer: Candidates should explain that creating a functional interface involves defining an interface with a single abstract method that represents the function's signature. They should discuss the @FunctionalInterface annotation and its optional use to enforce the single abstract method requirement. Candidates should provide an example of creating a custom functional interface.
Sample Answer: "To create a custom functional interface in Java, you define an interface with a single abstract method that represents the function you want to encapsulate. While it's not required, you can use the @FunctionalInterface annotation to explicitly designate your interface as functional and enforce the single abstract method requirement. Here's an example:
@FunctionalInterface
interface MyFunction {
void apply(int x, int y);
}
This interface defines a function that takes two integers as parameters and has no return type."
What to Look For: Seek candidates who can explain the process of creating a custom functional interface, including the role of the @FunctionalInterface annotation. They should understand the requirements for functional interfaces and be able to provide clear examples. Look for candidates who demonstrate creativity in designing functional interfaces for specific use cases.
These questions cover some of the fundamental concepts introduced in Java 8, including lambda expressions, default methods in interfaces, the Stream API, and functional interfaces. Candidates who can effectively answer these questions demonstrate a strong understanding of modern Java development techniques and are well-prepared for Java 8-focused interviews.
How to Answer: Candidates should explain that Optional is a container object that may or may not contain a non-null value. They should discuss how Optional is used to avoid NullPointerExceptions and to represent the possibility of a value being absent. Candidates should provide examples of using Optional for error handling and defensive programming.
Sample Answer: "The java.util.Optional class introduced in Java 8 is used to represent an optional value that may or may not be present. It helps in avoiding NullPointerExceptions by providing a way to handle potentially null values safely. Optional provides methods for checking the presence of a value, accessing the value if it's present, or providing a default value if it's absent. For example, Optional can be used to handle scenarios where a method may return null by wrapping the return value in an Optional."
What to Look For: Look for candidates who can articulate the purpose and usage of the Optional class for error handling. They should understand how Optional helps in writing more robust and expressive code by explicitly handling the absence of values. Strong candidates will provide examples demonstrating the use of Optional in different scenarios.
How to Answer: Candidates should explain that exceptions in Java 8 streams can be handled using the try-catch block within the stream pipeline or by using the Stream API's exception handling methods such as forEach
, map
, or flatMap
. They should discuss the implications of handling exceptions within a stream and the importance of maintaining stream immutability.
Sample Answer: "In Java 8 streams, exceptions can be handled using the try-catch block within the stream pipeline or by using the Stream API's exception handling methods such as forEach
, map
, or flatMap
. When handling exceptions within a stream, it's essential to consider the implications on stream immutability and ensure that the stream remains consistent. For example, if an exception occurs during a map operation, the stream may terminate prematurely, and subsequent operations may not be executed."
What to Look For: Seek candidates who can explain the various approaches to exception handling in Java 8 streams. They should understand the trade-offs involved in handling exceptions within a stream and be able to discuss best practices for maintaining stream integrity. Look for candidates who demonstrate awareness of potential pitfalls and strategies for robust error handling in stream operations.
How to Answer: Candidates should discuss the shortcomings of the legacy Date and Calendar classes and how the Date and Time API in Java 8 addresses these issues. They should highlight the immutability, thread-safety, and improved API design of the new Date and Time API. Candidates should provide examples of using the Date and Time API to perform common date and time operations.
Sample Answer: "The Date and Calendar classes in the Java standard library had several shortcomings, including mutability, lack of thread-safety, and poor API design. The Date and Time API introduced in Java 8 addresses these issues by providing immutable and thread-safe date and time classes with a more intuitive API. The new API allows for easier manipulation of dates, times, and time zones, and it supports modern date and time concepts such as periods, durations, and intervals."
What to Look For: Look for candidates who can articulate the advantages of the Date and Time API over the legacy Date and Calendar classes. They should demonstrate an understanding of the design principles behind the new API and its benefits in terms of immutability, thread-safety, and usability. Strong candidates will provide examples showcasing the use of the Date and Time API for common date and time operations.
How to Answer: Candidates should explain that parsing and formatting dates using the Date and Time API involves using the DateTimeFormatter
class to define custom date and time patterns. They should discuss the various predefined formatters available in the API and how to parse strings into LocalDate
, LocalTime
, or LocalDateTime
objects. Candidates should provide examples of parsing and formatting dates.
Sample Answer: "In Java 8's Date and Time API, parsing and formatting dates can be done using the DateTimeFormatter
class, which allows for defining custom date and time patterns. The API also provides several predefined formatters for common date and time formats. To parse a string into a LocalDate
, LocalTime
, or LocalDateTime
object, we use the parse()
method of the DateTimeFormatter
class. Similarly, to format a date or time object into a string, we use the format()
method."
What to Look For: Seek candidates who can explain the process of parsing and formatting dates using the Date and Time API in Java 8. They should be familiar with the DateTimeFormatter
class and its methods for parsing and formatting date and time objects. Look for candidates who can provide clear examples demonstrating the usage of the API for date and time manipulation.
How to Answer: Candidates should explain that CompletableFuture in Java 8 provides a way to perform asynchronous and non-blocking operations. They should discuss how CompletableFuture supports composition of asynchronous tasks, exception handling, and timeouts. Candidates should provide examples of using CompletableFuture for concurrent programming tasks.
Sample Answer: "CompletableFuture in Java 8 offers several advantages for concurrent programming. It allows us to perform asynchronous and non-blocking operations, enabling better utilization of system resources. CompletableFuture supports composition of asynchronous tasks, making it easy to chain multiple asynchronous operations together. Additionally, CompletableFuture provides built-in support for exception handling and timeouts, allowing for robust error handling in asynchronous code."
What to Look For: Look for candidates who can articulate the benefits of using CompletableFuture for concurrent programming tasks. They should understand how CompletableFuture simplifies asynchronous programming by providing features such as composition, exception handling, and timeouts. Strong candidates will be able to provide examples demonstrating the usage of CompletableFuture in real-world scenarios.
How to Answer: Candidates should explain that parallel streams in Java 8 are created by invoking the parallel()
method on a stream. They should discuss the implications of parallel stream processing, such as improved performance on multi-core processors. Candidates should provide examples of using parallel streams to parallelize computations.
Sample Answer: "To create and run a parallel stream in Java 8, we invoke the parallel()
method on a stream object. This instructs the Stream API to process the elements of the stream concurrently across multiple threads. Parallel streams are particularly useful for computationally intensive tasks that can benefit from parallelization, such as processing large collections or performing CPU-bound operations. Here's an example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.parallelStream()
.mapToInt(i -> i)
.sum();
This code snippet calculates the sum of integers in the list using a parallel stream."
What to Look For: Seek candidates who can explain how to create and run parallel streams in Java 8. They should understand the benefits and considerations of parallel stream processing, such as improved performance and potential thread-safety issues. Look for candidates who can provide examples demonstrating the usage of parallel streams for parallelizing computations.
How to Answer: Candidates should explain that default methods in interfaces cannot directly access private methods in Java 8. They should discuss the rationale behind this restriction and how it helps in maintaining encapsulation and preventing unintended dependencies. Candidates should provide alternative approaches for sharing code between default methods.
Sample Answer: "No, default methods in interfaces cannot directly access private methods in Java 8. This restriction is in place to enforce encapsulation and prevent unintended dependencies between default methods. Private methods in interfaces are meant to be implementation details and are not accessible outside the interface itself. If default methods need to share code, it's possible to extract common functionality into private helper methods within the same interface."
What to Look For: Look for candidates who can explain the accessibility rules for default and private methods in interfaces in Java 8. They should understand the importance of encapsulation and the rationale behind prohibiting direct access to private methods from default methods. Strong candidates will provide alternative strategies for sharing code between default methods within an interface.
How to Answer: Candidates should explain that conflicts arising from multiple default methods with the same signature in interfaces can be resolved by explicitly overriding the conflicting method in the implementing class. They should discuss the rules for method resolution and how to specify which default method implementation to use. Candidates should provide examples of resolving conflicts in interface inheritance.
Sample Answer: "When a class implements multiple interfaces with default methods having the same signature, a conflict arises, and the implementing class must provide its own implementation to resolve the conflict. This can be done by explicitly overriding the conflicting default method in the implementing class. Alternatively, the implementing class can choose to invoke a specific default method implementation using the interface name followed by the super
keyword. Here's an example:
interface InterfaceA {
default void foo() {
System.out.println("InterfaceA");
}
}
interface InterfaceB {
default void foo() {
System.out.println("InterfaceB");
}
}
class MyClass implements InterfaceA, InterfaceB {
@Override
public void foo() {
InterfaceA.super.foo(); // Explicitly choose InterfaceA's implementation
}
}
What to Look For: Seek candidates who can explain how conflicts arising from multiple default methods with the same signature in interfaces are resolved in Java 8. They should understand the rules for method resolution and be able to provide strategies for resolving conflicts, such as explicit method overriding or invocation of specific default method implementations. Look for candidates who can provide clear examples demonstrating the resolution of conflicts in interface inheritance.
How to Answer: Candidates should explain that memoization is a technique used to cache the results of expensive function calls to improve performance by avoiding redundant computations. They should discuss how memoization can be implemented using Java 8 features such as memoization with recursive functions or using memoization libraries like Guava. Candidates should provide examples demonstrating the implementation of memoization.
Sample Answer: "Memoization is a technique used to cache the results of expensive function calls to avoid redundant computations and improve performance. In Java 8, memoization can be implemented using features such as memoization with recursive functions or using memoization libraries like Guava. For example, we can implement memoization with recursive functions by storing previously computed results in a map and returning the cached result if available. This avoids recomputing the result for the same input."
What to Look For: Look for candidates who can explain the concept of memoization and its benefits in improving performance by caching function results. They should understand different approaches to implementing memoization in Java 8 and be able to provide examples demonstrating the implementation. Strong candidates will discuss the trade-offs involved in memoization and its applicability to different types of problems.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Preparing for a Java 8 interview requires a combination of technical proficiency, strategic planning, and effective communication. Let's delve into the essential steps you should take to ensure you're well-prepared for your Java 8 interview.
Before you step into the interview room, it's crucial to have a solid understanding of the company you're interviewing with and the technologies they use. Research the company's products, services, and industry verticals to gain insights into their business objectives and technical challenges. Familiarize yourself with their tech stack, paying close attention to any Java 8-related projects or initiatives they may have undertaken. By demonstrating a genuine interest in the company and aligning your skills with their needs, you'll set yourself apart as a candidate who's invested in the role.
While Java 8 introduces several new features, it's essential not to overlook the fundamentals of the Java programming language. Take the time to review core concepts such as object-oriented programming, inheritance, polymorphism, and exception handling. Ensure you're comfortable with topics like data structures, algorithms, and design patterns, as they form the foundation upon which Java 8 features are built. A solid grasp of fundamental Java concepts will not only help you answer interview questions more confidently but also enable you to apply Java 8 features effectively in real-world scenarios.
One of the most effective ways to prepare for a Java 8 interview is to practice coding exercises that specifically target Java 8 features. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of Java 8-specific challenges, including problems related to lambda expressions, streams, optional class usage, and functional interfaces. Dedicate time to solving these exercises, paying attention to both correctness and efficiency. Practice writing clean, idiomatic code that leverages Java 8 features to their fullest potential. By honing your problem-solving skills in the context of Java 8, you'll build confidence and competence in applying these features in a real-world setting.
In addition to technical proficiency, interviewers often assess candidates' familiarity with common Java 8 interview questions. Take the time to familiarize yourself with these questions, covering topics such as lambda expressions, stream API operations, optional class usage, default methods in interfaces, and functional interfaces. Practice articulating your responses clearly and concisely, providing examples where applicable. Remember that interview questions may vary depending on the role and the interviewer's preferences, so it's essential to be adaptable and prepared for a range of scenarios. By anticipating and practicing responses to common Java 8 interview questions, you'll be better equipped to showcase your expertise and problem-solving abilities during the interview.
In Java 8 interviews, candidates are often tested on their understanding and proficiency in utilizing key features introduced in Java 8. Let's explore these common topics in detail.
Lambda expressions are a cornerstone of Java 8, enabling developers to write more concise and expressive code. A lambda expression is essentially an anonymous function that can be used to represent a block of code. They are particularly useful when working with functional interfaces, as they allow you to pass behavior as a method argument. Understanding lambda expressions involves grasping concepts such as syntax, parameter types, and method references. Interview questions related to lambda expressions may include:
The Stream API introduced in Java 8 revolutionized the way developers work with collections. Streams provide a fluent and declarative way to process collections of objects, allowing for functional-style operations such as filtering, mapping, and reducing. Mastery of the Stream API involves understanding intermediate and terminal operations, parallel processing, and stream pipelines. Interview questions related to the Stream API may include:
The Optional class was introduced in Java 8 to address the problem of null references and NullPointerExceptions. It provides a container for an object that may or may not be present, allowing developers to write more robust and null-safe code. Understanding the Optional class involves knowing how to create and manipulate Optional objects, as well as best practices for using them effectively. Interview questions related to the Optional class may include:
orElse
, orElseGet
, and orElseThrow
.Java 8 introduced the concept of default methods in interfaces, allowing interfaces to have method implementations. This feature enables interface evolution without breaking existing implementations and facilitates multiple inheritance in Java. Understanding default methods involves knowing how to define and use them in interfaces, as well as their impact on existing code and interface design. Interview questions related to default methods may include:
Functional interfaces are interfaces that contain exactly one abstract method, serving as the foundation for lambda expressions and method references in Java 8. Mastery of functional interfaces involves understanding their role in functional programming, as well as popular functional interfaces provided by the Java API. Interview questions related to functional interfaces may include:
@FunctionalInterface
annotation and its significance.Predicate
, Consumer
, Function
, and Supplier
.
In addition to the fundamental concepts covered earlier, Java 8 interviews may delve into more advanced topics that demonstrate a candidate's depth of understanding and ability to apply Java 8 features in complex scenarios. Let's explore these advanced topics in detail.
Method references provide a concise way to refer to methods or constructors using a shorter syntax. They are particularly useful when working with lambda expressions and functional interfaces, allowing developers to reuse existing methods without writing additional code. Understanding method references involves grasping the different types of method references and their syntax. Interview questions related to method references may include:
CompletableFuture is a powerful feature introduced in Java 8 for asynchronous programming. It represents a future result of an asynchronous computation and allows developers to chain multiple asynchronous operations together. Mastery of CompletableFuture involves understanding its methods, handling exceptions, and leveraging its composability features. Interview questions related to CompletableFuture may include:
Java 8 introduced a new Date and Time API to address the shortcomings of the existing Date and Calendar classes. The Date and Time API provides a more comprehensive and flexible way to work with dates, times, and intervals. Understanding the Date and Time API involves familiarizing yourself with its classes, methods, and formatting options. Interview questions related to the Date and Time API may include:
These advanced Java 8 topics demonstrate your proficiency in leveraging the full potential of Java 8 features and showcase your ability to solve complex problems using modern Java programming techniques. By mastering these topics, you'll position yourself as a top-tier candidate for Java 8-related roles.
Preparing for a Java 8 interview goes beyond just technical knowledge. Here are some practical tips to help you succeed:
By following these practical tips, you'll be well-prepared to ace your Java 8 interview and showcase your skills and expertise effectively. Remember to approach the interview with confidence, enthusiasm, and a willingness to learn.
Mastering Java 8 interview questions is essential for both employers and candidates in today's software development landscape. Employers seek candidates who can leverage Java 8's powerful features to drive innovation and build scalable solutions. For candidates, Java 8 proficiency opens doors to exciting career opportunities and advancement. By understanding the fundamentals, practicing coding exercises, and familiarizing oneself with common interview topics, success in Java 8 interviews is within reach.
Remember, preparation is key. Stay updated with the latest Java 8 developments, build projects to solidify your skills, and practice effective communication during interviews. With dedication and practice, you'll be well-equipped to tackle any Java 8 interview with confidence and proficiency, paving the way for a successful career in software development.