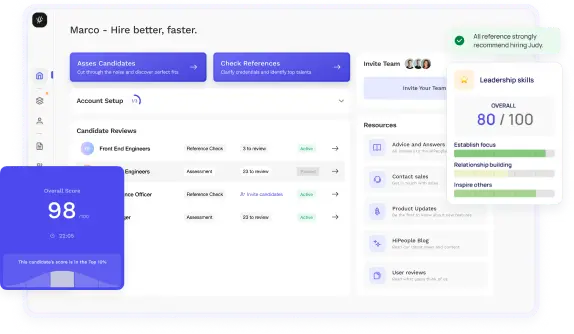
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
What’s the best way to assess a developer’s ability to create efficient, maintainable, and scalable software? The answer often lies in Object-Oriented Programming (OOP) interview questions. These questions help employers evaluate a candidate’s understanding of key programming principles like classes, objects, inheritance, and polymorphism, which are crucial for designing high-quality systems. By testing both theoretical knowledge and practical application, OOP interviews provide a clear picture of a developer’s ability to tackle complex challenges and contribute to building robust software.
Interviews can feel like a shot in the dark... unless you have the right data to guide you. Why waste time with generic questions and gut-feel decisions when you can unlock precision hiring with HiPeople?
Here’s how HiPeople changes the game:
Ready to transform your hiring process? 🚀 Book a demo today and see how HiPeople makes your interviews smarter, faster, and way more effective!
Object-Oriented Programming (OOP) is one of the most fundamental paradigms in software development today. It structures software in a way that organizes code around objects, which encapsulate both data and behaviors. OOP allows developers to model real-world scenarios more intuitively, making it easier to design, maintain, and scale complex systems. The principles of OOP—such as classes, inheritance, polymorphism, and encapsulation—enable developers to create code that is modular, reusable, and easier to debug.
OOP has become a cornerstone of modern software development because it promotes clear organization, flexibility, and maintainability. It supports the development of large, complex applications by breaking them down into smaller, manageable objects. This approach simplifies coding, testing, and debugging, and makes software more adaptable to changing requirements. Understanding how to use OOP effectively is essential for developers who want to build robust software and take part in modern development workflows.
At its core, Object-Oriented Programming is a way of organizing code that focuses on "objects." These objects are instances of classes, which define their structure and behavior. Each object holds data in the form of attributes (also called fields) and defines behavior through methods (functions or procedures).
OOP was designed to address the challenges of procedural programming, where data and behavior were often separate, leading to code that was harder to maintain and scale. The introduction of classes and objects in OOP allows developers to bundle both data and the actions that manipulate that data, making the code more cohesive and organized.
OOP uses four key principles:
OOP can be applied in many different programming languages, with each language offering its own syntax and mechanisms for defining and managing classes and objects. While the specific implementation of OOP may vary, the foundational principles remain the same.
Object-Oriented Programming is not just a theoretical concept—it's a practical tool that plays a crucial role in software development. In any software engineering role, understanding how to effectively use OOP principles can have a significant impact on both the quality and maintainability of the code you produce. Here’s why OOP is so important in modern software engineering:
OOP interviews are an essential part of the hiring process for software engineering roles because they provide a way to assess both a candidate’s technical proficiency and their ability to think through complex software design problems. Here's why they matter from both perspectives:
OOP interviews allow both employers and candidates to gauge a candidate's technical competence, problem-solving abilities, and design skills, making them an essential part of the hiring process in modern software engineering roles. They help ensure that the right person is selected for the job, with the technical foundation needed to succeed in a fast-paced development environment.
How to Answer: When answering this question, candidates should demonstrate an understanding of the core principles of OOP: Encapsulation, Inheritance, Polymorphism, and Abstraction. It’s important to explain not just the terminology, but also how these concepts help in writing maintainable and scalable code.
Sample Answer: "The four pillars of Object-Oriented Programming are encapsulation, inheritance, polymorphism, and abstraction. Encapsulation refers to bundling the data and methods that operate on the data within a single unit or class, restricting direct access to some of the object's components. Inheritance allows a class to inherit properties and methods from another class, promoting code reuse. Polymorphism enables objects of different classes to be treated as objects of a common superclass, simplifying code and making it more flexible. Abstraction hides the complex implementation details and shows only the essential features of an object, making it easier to interact with objects without worrying about their inner workings."
What to Look For: Look for candidates who provide clear and comprehensive explanations of each pillar, demonstrating their understanding of how they contribute to software design. Avoid candidates who focus on just one or two pillars, or who cannot explain their application in real-world scenarios.
How to Answer: Candidates should be able to explain encapsulation by describing how it helps in protecting an object's state by controlling access to its internal data. Look for an example where getters and setters are used to manage private fields.
Sample Answer: "Encapsulation is the practice of keeping the internal workings of an object hidden from the outside world. This is achieved by making the object’s attributes private and providing public methods to access and update them. For example, in a BankAccount
class, the balance could be private, and we can provide methods like deposit()
and withdraw()
to modify the balance. This ensures that the balance cannot be directly modified from outside the class, preventing invalid or unwanted changes."
What to Look For: A strong answer should include an example where private data is accessed through getter and setter methods. Watch for answers where candidates fail to distinguish between public and private access modifiers or don't provide a clear, practical example.
How to Answer: Candidates should be able to distinguish between the two concepts. Overloading involves creating multiple methods with the same name but different parameters, while overriding involves a subclass providing its specific implementation of a method defined in its superclass.
Sample Answer: "Method overloading occurs when a class has more than one method with the same name but different parameters (either in number or type). For example, a print()
method might accept different types of parameters: one that accepts a string and another that accepts an integer. On the other hand, method overriding occurs when a subclass provides its implementation of a method that is already defined in the superclass, using the same method signature. This is useful for providing specific behavior in the subclass. For example, a Dog
class might override a makeSound()
method from an Animal
superclass to provide a dog-specific implementation, such as barking."
What to Look For: Look for a clear distinction between overloading and overriding. Candidates should be able to demonstrate both concepts with real-world examples. Candidates who mix the two concepts or provide inaccurate examples may lack a fundamental understanding of OOP principles.
How to Answer: This question tests the candidate's ability to explain one of the primary benefits of inheritance in OOP. They should be able to articulate how inheritance allows subclasses to inherit properties and behaviors from parent classes, reducing redundancy and promoting efficient code reuse.
Sample Answer: "Inheritance promotes code reusability by allowing a subclass to inherit fields and methods from a parent class, which reduces the need for writing redundant code. For instance, if you have a Vehicle
class with a start()
method, and you want to create a Car
class, instead of writing the start()
method again for the Car
class, the Car
class can simply inherit from the Vehicle
class and use its start()
method. This not only saves time but also makes the code more maintainable and easier to extend."
What to Look For: A good answer should focus on the concept of reducing redundancy and promoting maintainability. Watch for candidates who either fail to explain the concept clearly or focus solely on the theoretical aspects without giving a practical example.
How to Answer: Candidates should explain the role of abstract classes in defining a common interface while leaving the implementation to subclasses. They should also note that abstract classes can contain both abstract methods (without implementation) and concrete methods (with implementation).
Sample Answer: "An abstract class is a class that cannot be instantiated on its own and serves as a blueprint for other classes. The main purpose of an abstract class is to provide a common base that subclasses can inherit from. It may include abstract methods, which must be implemented by subclasses, as well as concrete methods that can be inherited directly. For example, in a Shape
class, you could have an abstract method draw()
, which would be implemented by subclasses like Circle
and Rectangle
. The abstract class ensures that all shapes have a draw()
method but allows the specific details of how the method works to vary across different shapes."
What to Look For: The ideal answer will show an understanding of the purpose of abstract classes and how they differ from regular classes. Candidates should be able to explain abstract methods and provide a meaningful example. Beware of candidates who cannot differentiate between abstract classes and interfaces.
How to Answer: Candidates should clarify that an interface defines a contract with no implementation, while an abstract class can contain both abstract and concrete methods. An interface is used for achieving polymorphism, while an abstract class is often used for sharing common functionality.
Sample Answer: "An interface in OOP is a contract that defines a set of methods that must be implemented by any class that implements the interface. Interfaces only declare method signatures without providing implementation. In contrast, an abstract class can contain both abstract methods, which must be implemented by subclasses, and concrete methods with actual implementation. A key difference is that a class can implement multiple interfaces but can only inherit from one abstract class. For example, an Account
interface might have methods like deposit()
and withdraw()
, and classes like BankAccount
and CreditAccount
would implement these methods. An abstract class, like Vehicle
, may have a concrete method start()
, while subclasses like Car
and Bike
would override specific methods like drive()
."
What to Look For: The answer should clearly explain the difference between interfaces and abstract classes. Candidates should also provide concrete examples. Be cautious of vague answers that don’t highlight key distinctions or provide poor examples.
How to Answer: Candidates should discuss principles like SOLID principles, the use of design patterns, and modularity. They should demonstrate an understanding of writing clean, well-structured code that can easily be modified or extended in the future.
Sample Answer: "To ensure scalability and maintainability, I focus on following SOLID principles: single responsibility, open/closed, Liskov substitution, interface segregation, and dependency inversion. These principles help in creating a design that is modular, easy to understand, and can be extended without modifying existing code. Additionally, I use design patterns like Factory or Observer to manage object creation and communication, making the code more flexible and less coupled. Finally, I ensure that the code is well-documented and tested, which simplifies future modifications and improves maintainability."
What to Look For: Look for candidates who demonstrate a deep understanding of design principles and practical experience applying them. Strong candidates will be able to describe how they’ve applied these concepts in real-world scenarios. Watch for candidates who can’t explain how to make their designs scalable or who overlook key principles like modularity or testing.
How to Answer: Candidates should explain that dependency injection is a technique for achieving Inversion of Control (IoC), where objects are provided their dependencies rather than creating them directly. This promotes decoupling and makes the code more flexible and easier to test.
Sample Answer: "Dependency injection is a design pattern where objects receive their dependencies from an external source, rather than creating them themselves. This helps in decoupling the components, making the system more flexible and easier to test. For instance, if a class Car
depends on an Engine
class, instead of the Car
class creating an Engine
object, it would receive the Engine
object through its constructor or a setter method. This way, we can easily swap out the Engine
for a different implementation or mock the Engine
in unit tests without changing the Car
class."
What to Look For: Look for candidates who can explain the core benefits of dependency injection, such as decoupling and flexibility. Strong answers will also discuss how dependency injection makes testing easier. Avoid candidates who fail to explain the practical benefits or miss the key point of externalizing dependencies.
How to Answer: Candidates should be able to explain that composition involves building classes using other objects, while inheritance is a relationship between classes where one class inherits the properties and methods of another.
Sample Answer: "Composition and inheritance are two different approaches for creating relationships between classes. Inheritance is when a subclass derives from a superclass and inherits its properties and behaviors. For example, a Car
class might inherit from a Vehicle
class. Composition, on the other hand, is when one class contains an instance of another class to achieve functionality. For instance, a Car
class may have a Engine
object, and the Car
class delegates certain behaviors, like starting the engine, to the Engine
class. Composition is often preferred over inheritance because it promotes greater flexibility and reduces the risks of creating tight coupling."
What to Look For: A strong response should provide a clear distinction between composition and inheritance and include examples that demonstrate the advantages of composition. Candidates should highlight flexibility and the ability to modify objects more easily through composition.
How to Answer: Candidates should define design patterns as reusable solutions to common design problems. They should be able to provide an example of a specific design pattern they’ve used and explain how it helped solve a particular problem.
Sample Answer: "A design pattern is a proven, reusable solution to a common problem that arises during software design. One design pattern I’ve used extensively is the Singleton pattern, which ensures that a class has only one instance and provides a global point of access to that instance. For example, I used the Singleton pattern to manage the database connection in a project. By making the connection class a Singleton, I ensured that only one connection was open at any time, which helped with resource management and made the code more efficient and easy to maintain."
What to Look For: Look for candidates who can articulate the purpose and benefit of design patterns. Strong candidates will give specific examples of patterns they’ve used and explain how it contributed to a successful design. Watch for candidates who cannot provide a meaningful example or fail to explain the impact of the pattern.
How to Answer: Candidates should explain that code refactoring involves restructuring existing code to improve its readability, performance, and maintainability without changing its external behavior. They should highlight the importance of refactoring to reduce technical debt and make the system more adaptable.
Sample Answer: "Code refactoring is an essential part of maintaining a healthy codebase. It’s important to refactor periodically to reduce technical debt, improve readability, and ensure scalability. For example, I might refactor redundant code by breaking it into smaller, more manageable methods or classes. I also focus on improving the design, such as eliminating large, monolithic classes by introducing interfaces or applying the SOLID principles. Refactoring should always be accompanied by thorough testing to ensure that no existing functionality is broken."
What to Look For: A good answer will reflect an understanding of refactoring principles, such as simplifying code and reducing complexity. Look for candidates who mention tools, techniques, or specific methods used for refactoring, such as code reviews or automated testing to ensure refactoring doesn't introduce bugs.
How to Answer: Candidates should be able to describe at least a few commonly used design patterns like Singleton, Factory, and Observer, explaining when and why they are appropriate in object-oriented systems.
Sample Answer: "Some common design patterns include the Singleton pattern, which ensures that only one instance of a class exists; the Factory pattern, which helps in creating objects without specifying the exact class of object that will be created; and the Observer pattern, which is used to create a subscription model to notify multiple objects about state changes. These patterns help solve common problems in object-oriented systems, such as ensuring a class has a single instance or managing dependencies in a flexible way."
What to Look For: A strong candidate will mention at least two or three design patterns, offering context about their real-world application. The candidate should show an understanding of why each pattern is useful in the context of object-oriented design.
How to Answer: Candidates should talk about optimizing system performance at various levels, such as minimizing object creation, caching, and reducing memory usage. They should also mention profiling tools for identifying performance bottlenecks.
Sample Answer: "Optimizing performance in an object-oriented system often involves reducing unnecessary object creation and leveraging techniques like object pooling. For example, rather than creating new objects repeatedly in a loop, I’d use object pooling to reuse existing instances, which can greatly reduce memory overhead. Additionally, I would consider caching frequently accessed data to minimize recalculating or fetching it from a database or network. Profiling tools like JProfiler or VisualVM can be used to identify memory leaks and optimize memory usage."
What to Look For: Look for candidates who focus on both object-level optimizations and broader system-wide improvements. Strong answers should include specific techniques and tools that the candidate has used to optimize performance.
How to Answer: Candidates should explain their understanding of memory management in object-oriented programming, focusing on automatic garbage collection mechanisms in languages like Java or C#. They should also discuss how to minimize memory leaks and improve memory usage efficiency.
Sample Answer: "In languages with automatic garbage collection like Java or C#, memory management is largely handled by the runtime environment, but developers still need to be mindful of memory usage. For example, I avoid holding references to objects that are no longer needed to allow the garbage collector to reclaim memory. I also make sure to nullify references in collections or caches once they’re no longer in use. In cases where memory management is more manual, like in C++, I focus on proper use of new
and delete
to prevent memory leaks."
What to Look For: A good response will demonstrate knowledge of how garbage collection works in the context of OOP, as well as strategies for managing memory efficiently. Candidates should show awareness of both automatic and manual memory management techniques.
How to Answer: Candidates should focus on writing clean, understandable code that follows established coding conventions, along with strategies for testing and documentation. They should also mention using version control and code reviews.
Sample Answer: "To ensure code maintainability, I focus on writing clean, well-documented code that follows best practices and industry standards. I ensure that code is modular by using small, reusable functions and classes. I also follow SOLID principles to ensure that the code remains flexible and can be easily modified without introducing bugs. Additionally, I write unit tests to catch regressions and make the code easier to refactor in the future. Regular code reviews and using version control systems like Git also help ensure that the codebase remains in good shape."
What to Look For: A strong answer will highlight the importance of clean code, testing, and good documentation. Candidates should mention tools like version control systems and the role of code reviews in maintaining high code quality.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Before heading into an OOP interview, it’s crucial to grasp the fundamental concepts that will be tested. OOP revolves around structuring code in a way that is modular, reusable, and maintainable. These concepts not only shape the way you write code but also influence how you approach solving problems in a scalable way. Let's break down the essential topics that you must have a solid understanding of.
Classes and objects are at the core of Object-Oriented Programming. Understanding these concepts allows you to build the fundamental building blocks of your program, such as defining the structure and behavior of entities in your code.
A class is essentially a template or blueprint for creating objects. It defines the properties (often called attributes or fields) and methods (functions) that the object will have. A class allows you to bundle data and the methods that operate on that data in one place.
An object, on the other hand, is an instance of a class. It is created based on the class blueprint and represents a specific entity in the program. Each object can have different data but will share the methods and attributes defined in the class.
For example, think about a Car
class that can represent a real-world car. You might have properties like make
, model
, and year
that define the car's attributes, and methods like start()
and stop()
that define actions the car can perform. Each specific car in the real world would be an object created from the Car
class, with unique values for those properties.
Here’s how this looks in code:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def start(self):
print(f"{self.make} {self.model} is starting!")
def stop(self):
print(f"{self.make} {self.model} is stopping!")
# Create an instance of the Car class
car1 = Car("Toyota", "Corolla", 2020)
car1.start() # Outputs: Toyota Corolla is starting!
These four principles form the foundation of OOP. They help developers create flexible, modular, and scalable code by promoting reusability, data protection, and clearer design.
Inheritance allows you to create new classes that are based on existing ones. This is a way of reusing code and making your classes more flexible. When one class inherits from another, the child class gets access to the properties and methods of the parent class but can also have additional features or override existing methods.
For instance, if you have a Vehicle
class with common properties like make
, model
, and year
, and methods like start()
and stop()
, you can create more specialized classes like Car
and Truck
, which inherit from Vehicle
but add their own specific attributes or behaviors.
class Vehicle:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def start(self):
print(f"{self.make} {self.model} is starting!")
class Car(Vehicle):
def __init__(self, make, model, year, doors):
super().__init__(make, model, year) # Call the parent class constructor
self.doors = doors
def open_doors(self):
print(f"Opening {self.doors} doors")
# Instantiate the Car class
car1 = Car("Toyota", "Corolla", 2020, 4)
car1.start()
car1.open_doors()
Encapsulation refers to the practice of keeping data private within a class and providing access to it only through public methods (getters and setters). This helps to protect the internal state of an object from unwanted changes and promotes data integrity.
You encapsulate data in a class by using private variables (often prefixed with an underscore in Python) and public methods to interact with those variables. This gives you control over how the data is accessed and modified.
class Account:
def __init__(self, balance):
self._balance = balance # _balance is private
def deposit(self, amount):
if amount > 0:
self._balance += amount
def withdraw(self, amount):
if 0 < amount <= self._balance:
self._balance -= amount
def get_balance(self):
return self._balance
# Using the Account class
account = Account(1000)
account.deposit(500)
account.withdraw(200)
print(account.get_balance()) # Outputs: 1300
Encapsulation ensures that you don’t allow clients to access and change your internal state in ways that could lead to errors or inconsistent behavior.
Polymorphism allows objects of different classes to be treated as instances of the same class through inheritance. The most common way this is implemented is through method overriding and interfaces.
In OOP, polymorphism can be achieved by having a method in a parent class that is overridden by methods in child classes. This allows you to call the same method on different objects, but each object will respond in its own way, depending on its class.
Here’s a simple example of polymorphism using method overriding:
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof"
class Cat(Animal):
def speak(self):
return "Meow"
# Instantiate Dog and Cat objects
dog = Dog()
cat = Cat()
# Polymorphism: the same method name calls different implementations
print(dog.speak()) # Outputs: Woof
print(cat.speak()) # Outputs: Meow
Abstraction is the practice of hiding complex implementation details and exposing only the essential features of an object. It allows you to work with higher-level objects without worrying about the underlying details. You can achieve abstraction through abstract classes or interfaces, which define a contract but leave the implementation to the subclasses.
Abstract classes contain abstract methods—methods without a body—that must be implemented by any concrete (non-abstract) subclass.
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * (self.radius ** 2)
circle = Circle(5)
print(circle.area()) # Outputs: 78.5
Abstraction simplifies interaction with complex systems and allows developers to focus on high-level functionality rather than low-level details.
Design patterns are proven, reusable solutions to common software design problems. They provide a standard way of solving a particular problem that has been tested and refined by the programming community over time. Understanding common design patterns is essential for writing effective OOP code, as they can help you structure your application to be more maintainable, scalable, and understandable.
Some of the most widely used OOP design patterns include:
In an interview, understanding design patterns can help you demonstrate your ability to structure code efficiently, address problems using industry-standard practices, and communicate your design decisions effectively.
Exception handling is an important aspect of building robust software. In OOP, exceptions are used to handle runtime errors that can occur during program execution. Properly handling exceptions ensures that your application doesn’t crash unexpectedly and allows you to deal with errors gracefully.
In OOP, exceptions are typically raised using the raise
keyword and caught with try
and except
blocks. This helps you maintain control over how errors are handled and enables you to present meaningful error messages or fallback solutions.
class Division:
def divide(self, a, b):
try:
result = a / b
except ZeroDivisionError:
print("Cannot divide by zero!")
else:
return result
div = Division()
print(div.divide(10, 2)) # Outputs: 5.0
print(div.divide(10, 0)) # Outputs: Cannot divide by zero!
In this example, the division operation is attempted inside a try
block. If there’s an error (in this case, a division by zero), the except
block will handle it, preventing the program from crashing. Handling exceptions is crucial for making your code reliable and user-friendly.
By mastering these core concepts—classes and objects, inheritance, encapsulation, polymorphism, abstraction, design patterns, and exception handling—you’ll be well-prepared to demonstrate your OOP expertise in any interview setting.
OOP interviews vary significantly depending on the candidate's experience level and the role they’re applying for. Understanding what to expect based on your job level is essential for tailoring your preparation. Whether you're a junior developer just starting out or a senior developer aiming to design large-scale systems, each role will have specific expectations regarding your knowledge and application of OOP concepts. Let’s explore what you need to know for different positions.
As a junior developer, your main focus will be on demonstrating your understanding of the core OOP principles and how you apply them in simple scenarios. You will not be expected to have extensive experience designing large systems but should be comfortable with foundational concepts and basic coding tasks.
For a junior role, you can expect interview questions that cover the basic building blocks of OOP, such as:
Here’s an example of the types of questions that might be asked during a junior-level OOP interview:
The goal in these interviews is to ensure that you understand basic concepts and can apply them in straightforward coding problems. Your ability to solve small coding challenges with these OOP principles will be a key focus.
As a mid-level developer, you will be expected to apply more advanced OOP concepts to solve complex problems. The focus here shifts from simply knowing the fundamentals to demonstrating your ability to use those principles to build maintainable, scalable applications. You may encounter scenarios where you need to design more intricate systems or refactor existing code to be more efficient and reusable.
Here are some advanced topics you might be tested on:
Here are a few potential questions you might face at the mid-level:
Expect to demonstrate how you apply your understanding of OOP concepts to real-world software development scenarios. The ability to balance good code structure with practical problem-solving will be essential.
For senior developers, interviews are less about technical knowledge and more about strategic thinking and system design. You will be expected to demonstrate your ability to design scalable, efficient, and maintainable systems. OOP principles will still be at the heart of your design, but you’ll be applying them at a much larger scale, often involving multiple components or services.
Some of the areas of focus for senior-level interviews include:
Sample senior developer interview questions might include:
You’ll be expected to show that you not only understand OOP concepts but can also apply them to solve complex, real-world problems with an eye toward future growth.
When you're at the architect level, you are expected to think about the big picture. Your job is to design the overarching structure of software systems, ensuring they are well-integrated, scalable, and maintainable. Architects must balance technical knowledge with strategic thinking, aligning the architecture with business goals and user needs.
As an architect, you will work on:
Possible architect-level interview questions might include:
As an architect, you’ll be expected to bring together a comprehensive understanding of OOP concepts, system design principles, and real-world business needs to create efficient, long-lasting solutions.
Object-Oriented Programming (OOP) is foundational to many of the most widely used programming languages and frameworks today. Its principles have been integrated into various languages and development environments to improve the modularity, scalability, and maintainability of code. Whether you are using Java, C#, Python, or other languages, OOP plays a crucial role in organizing and structuring code to handle complex systems. Understanding how OOP is utilized in these languages and frameworks is essential for both developers and employers when creating modern applications.
OOP is not confined to a single programming language but is a core feature in many popular ones. The way OOP is implemented may vary slightly between languages, but the core principles remain the same: classes, objects, inheritance, encapsulation, polymorphism, and abstraction. Let’s explore how OOP is used in some of the most common programming languages.
Java is one of the most well-known and widely used programming languages, and it has a strong emphasis on OOP. Everything in Java is treated as an object, and even primitive types (like integers) are encapsulated into objects using wrapper classes.
private
, protected
, and public
being used to control the visibility and accessibility of variables and methods.C# is another language that heavily relies on OOP principles, with a strong emphasis on object-oriented programming practices in enterprise software development.
Python is a highly flexible language that supports multiple programming paradigms, including OOP. While Python is not as rigidly object-oriented as Java or C#, it still offers strong support for OOP principles.
Frameworks built on top of these programming languages are designed to streamline the development process and increase efficiency by offering pre-built tools and libraries that follow OOP principles. These frameworks abstract away much of the repetitive boilerplate code, allowing developers to focus on business logic instead of reinventing the wheel.
For example, frameworks like Spring (Java), .NET (C#), and Django (Python) are all structured around OOP principles, enabling developers to build robust and maintainable applications more efficiently.
Spring is an open-source framework for building Java-based enterprise applications. It uses OOP principles to enable developers to create modular and loosely coupled systems, making it easier to maintain and scale applications.
The .NET framework is a comprehensive development platform that supports various programming languages, including C#. It uses OOP principles to create modular and reusable components, which help developers build applications faster.
Django is a high-level Python framework that enables fast development of secure and maintainable websites. It uses the principles of OOP to help developers quickly build applications while adhering to best practices.
While OOP has dominated the software development landscape for decades, functional programming (FP) is gaining popularity due to its emphasis on immutability, first-class functions, and statelessness. Many modern programming languages and frameworks allow developers to combine both OOP and FP techniques, creating hybrid approaches that take advantage of the strengths of both paradigms.
OOP and functional programming are often seen as two opposing paradigms, but the truth is that they can complement each other in powerful ways. Let's look at how these two paradigms can work together.
Java is primarily an object-oriented language, but starting with Java 8, functional programming features were introduced. This includes lambda expressions, streams, and functional interfaces, which enable developers to apply functional programming principles alongside object-oriented designs.
C# is another language that supports both OOP and functional programming, enabling developers to choose the best approach depending on the situation.
Python, with its flexible nature, allows developers to mix OOP and functional programming styles seamlessly. This flexibility makes it an ideal language for exploring the synergies between the two paradigms.
While OOP is excellent for creating modular and reusable components, functional programming brings a new perspective by focusing on immutability and statelessness. Combining both paradigms gives developers the flexibility to solve problems using the most appropriate tool for the job, making modern development frameworks and languages much more powerful and versatile.
By blending OOP and FP, you can create more expressive, maintainable, and efficient code while maintaining the scalability and robustness of traditional object-oriented design. This hybrid approach is becoming increasingly common in the development world, and understanding how to bridge the gap between these two paradigms will give you an edge as a developer.
Preparing for an OOP interview requires a solid understanding of key concepts, as well as the ability to demonstrate how you can apply them to solve real-world problems. Being able to articulate your thought process and reason about design decisions is just as important as writing clean, functional code. Here are some essential tips to help you excel in OOP interviews.
By focusing on these areas, you'll be well-equipped to handle OOP interview challenges with confidence.
While technical interviews provide insight into a candidate’s OOP knowledge, there are additional ways to assess a developer’s proficiency with OOP principles and their ability to work effectively in real-world scenarios. Beyond the interview itself, there are several methods to evaluate how well a candidate truly understands and applies OOP in the context of their day-to-day work.
By using these methods, you can better evaluate a candidate's true expertise in OOP, ensuring they can apply their knowledge effectively in practical, real-world situations.
Mastering OOP interview questions is essential for any developer who wants to succeed in the fast-paced world of software engineering. These questions test more than just your ability to write code—they evaluate how well you can apply core principles like inheritance, encapsulation, and polymorphism to solve real-world problems. Whether you're just starting out or you're an experienced developer, the ability to think through problems, design systems, and communicate your approach clearly is critical. OOP isn’t just a set of abstract concepts; it’s a practical tool that helps you build software that is scalable, maintainable, and adaptable to changing needs.
As an employer, knowing how to evaluate OOP skills in an interview setting ensures that you’re hiring candidates who can contribute to your team’s success from day one. By focusing on both theoretical knowledge and practical application, OOP interview questions provide insight into how a candidate will approach complex development tasks, work with teams, and create high-quality code. For candidates, understanding the depth and scope of these questions can help you prepare effectively, ensuring you can demonstrate not just what you know, but how you can apply that knowledge to solve problems and drive results.