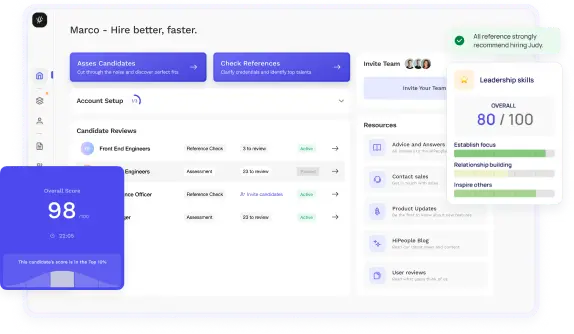
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you preparing for a TypeScript interview and wondering how to stand out? Navigating the world of TypeScript can be challenging, especially when it comes to showcasing your skills effectively. This comprehensive guide delves into everything you need to know about TypeScript interview questions, offering insights into core concepts, advanced topics, and practical scenarios. Whether you’re a candidate aiming to demonstrate your expertise or an employer seeking to assess proficiency accurately, this guide provides valuable information to help you excel. From understanding TypeScript’s role in modern development to tackling complex interview questions, you’ll find detailed explanations and practical tips to enhance your preparation and performance.
TypeScript is a powerful superset of JavaScript that introduces static typing and additional features to enhance the development process. Developed and maintained by Microsoft, TypeScript builds on JavaScript by adding type annotations, which allow developers to catch errors early and improve code reliability. As a compiled language, TypeScript is transformed into plain JavaScript, making it compatible with all JavaScript environments. This transformation provides a way to use TypeScript’s advanced features while still running the code on any platform that supports JavaScript.
TypeScript enhances JavaScript by offering:
TypeScript’s design makes it particularly well-suited for large-scale applications and complex codebases, where maintaining type safety and clarity is crucial.
TypeScript has gained significant traction in modern software development due to its ability to address common challenges faced by developers working with JavaScript. Its relevance is highlighted by several key factors:
By addressing issues related to code quality, scalability, and productivity, TypeScript has become a crucial tool in modern development environments.
TypeScript interviews are essential for assessing a candidate’s proficiency and understanding of the language. These interviews play a critical role in evaluating skill levels by focusing on various aspects of TypeScript expertise:
By conducting thorough TypeScript interviews, employers can better understand a candidate’s technical abilities and suitability for roles that require advanced TypeScript skills.
How to Answer: Begin by explaining that TypeScript is a superset of JavaScript that primarily adds static types to the language. Emphasize the advantages of TypeScript, such as better tooling, advanced features like interfaces and enums, and the ability to catch errors at compile time. Highlight how these features can lead to more maintainable and scalable code.
Sample Answer: "TypeScript is a statically typed superset of JavaScript that compiles to plain JavaScript. The main difference is that TypeScript allows us to use types, which provides better tooling support and catches potential errors during compile time rather than at runtime. This can significantly reduce the number of bugs in production, making it easier to maintain and scale applications."
What to Look For: Look for a candidate's clear understanding of TypeScript's purpose and differences from JavaScript. Strong candidates will articulate how TypeScript enhances code quality and productivity, while weaker candidates might struggle to differentiate between the two languages or focus only on syntax without discussing the benefits of type safety.
How to Answer: Discuss the concept of types in TypeScript, which can include primitives like string, number, and boolean, as well as more complex types such as arrays, tuples, enums, and interfaces. Provide examples for clarity, illustrating how these types improve code safety and readability.
Sample Answer: "In TypeScript, types are a way to specify what kind of values a variable can hold. For instance, we can declare a variable as a string: let name: string = 'Alice'; or as an array of numbers: let scores: number[] = [90, 85, 80];. We can also create our own types using interfaces, like interface Person { name: string; age: number; }, allowing us to define the shape of an object clearly."
What to Look For: Candidates should demonstrate a solid comprehension of basic TypeScript types and the benefits of using these types, such as reducing runtime errors. Watch for any misunderstandings about static versus dynamic typing and any inappropriate examples.
How to Answer: Explain that interfaces in TypeScript define the structure of an object and can be extended or implemented by classes. Mention that while both interfaces and types can describe object shapes, interfaces are more suited for declaring contracts in code, especially for objects and classes.
Sample Answer: "Interfaces in TypeScript are used to define the shape of objects, allowing you to specify what properties and methods an object should have. Unlike types, which are more flexible and can describe unions or other complex types, interfaces are specifically designed to create contracts for classes and can be extended or merged. For example, I can define an interface for a car: interface Car { make: string; model: string; year: number; }. Classes can then implement this interface to ensure they adhere to its structure."
What to Look For: Candidates should articulate the differences between interfaces and types, especially regarding functionality and use cases. Strong answers will note the ability of interfaces to be extended and the context in which one might be preferable over the other.
How to Answer: Define generics as a way to create reusable components that work with various data types without sacrificing type safety. Describe how generics allow for more flexible and dynamic code and provide a clear example, such as a generic function that can return different types based on the input.
Sample Answer: "Generics in TypeScript enable you to create components that can work with any data type while maintaining type safety. For example, I can define a generic function like: function identity<T>(arg: T): T { return arg; }, which returns whatever type of argument is passed. If I call identity<string>('hello'), the function knows to treat the input as a string. This approach enhances code reusability and helps avoid type-related errors."
What to Look For: Look for a clear understanding of generics and how they enhance code flexibility. A good candidate will provide a relevant example and explain the benefits of generics in making code safer and easier to maintain.
How to Answer: Discuss the role of the tsconfig.json file in a TypeScript project as it defines the compiler options and processing rules. Explain key options like compilerOptions, include, and exclude, and how they affect the compilation process and output directory.
Sample Answer: "The tsconfig.json file is crucial for managing TypeScript configurations in a project. It specifies various options for the compiler, such as compilerOptions, which can include flags like target to determine JavaScript version output, and module to specify the module system. Additionally, include and exclude help in defining which files should be compiled. For instance, I might set target: 'es6' to compile my TypeScript to ES6 JavaScript, ensuring compatibility with modern browsers."
What to Look For: Candidates should show knowledge of key compiler options and their implications on project behavior. Look for clarity in explaining how different configurations influence the compilation and execution of TypeScript code.
How to Answer: Explain these utility types, emphasizing their use cases in enhancing the flexibility and safety of TypeScript code. Describe how each utility type modifies an existing type, with examples illustrating their practical applications.
Sample Answer: "TypeScript provides several utility types that enhance the functionality of existing types. For example, Partial<T> makes all properties of type T optional, allowing for flexibility in object creation—useful when dealing with forms where not all fields need to be populated. Readonly<T> makes properties of type T immutable, preventing modifications after creation, which is beneficial for maintaining constant values. Lastly, Record<K, T> creates a type with keys of type K and values of type T, useful for constructing objects where keys are known beforehand."
What to Look For: Strong candidates will demonstrate familiarity with these utility types alongside their relevant applications. Look for examples that illustrate the functionality and benefits associated with each utility type.
How to Answer: Discuss methods like using DefinitelyTyped for existing types or creating custom type definitions using a declaration file (.d.ts). Emphasize the importance of contributing back to the community if the library is widely used.
Sample Answer: "When dealing with a third-party library without TypeScript support, I would first check DefinitelyTyped for existing type definitions. If none exist, I would create a declaration file, such as custom-lib.d.ts, where I define the types for the library functions and classes I plan to use. This approach allows me to maintain type safety in my application. Additionally, if my type definitions could benefit others, I would consider contributing them back to the community."
What to Look For: Look for a candidate's resourcefulness and understanding of the community-driven aspects of TypeScript. Strong candidates should show familiarity with both DefinitelyTyped and the process of creating custom type definitions.
How to Answer: Explain how TypeScript integrates with popular frameworks such as React or Angular, enhancing their capabilities by providing type safety and improved tooling. Mention specific features, such as typing props in React components or leveraging Angular decorators in TypeScript.
Sample Answer: "TypeScript works exceptionally well with frameworks like React and Angular. In React, I can define the types for props directly in the component, which provides enhanced autocompletion and error-checking during development. For instance, const MyComponent: React.FC<MyProps> = (props) => { ... }. In Angular, TypeScript’s decorators enhance classes, making it easier to define services, components, and modules while ensuring strict type checks throughout the application, greatly improving code reliability."
What to Look For: Candidates should demonstrate practical experience with TypeScript in conjunction with certain frameworks. They should be comfortable discussing real-world applications and how type safety boosts development efficiency and code quality.
How to Answer: Discuss how TypeScript’s static type-checking allows developers to catch potential issues during compilation rather than at runtime. Highlight how type annotations and interfaces contribute to writing more predictable and understandable code.
Sample Answer: "TypeScript significantly reduces runtime errors by providing static type-checking. As TypeScript enforces types during compile time, it surfaces potential type mismatches and logical errors early in the development process. For example, if I declare a variable as a string, attempting to assign a number will trigger an error, allowing me to catch it before it reaches runtime. This type safety not only mitigates bugs but also enhances code clarity for future maintenance."
What to Look For: Look for a clear understanding of how TypeScript's type system works in practice and how it contributes to error avoidance. Strong candidates will provide specific examples demonstrating this capability.
How to Answer: Talk about establishing a clear folder structure, defining boundaries between modules, and using interfaces to define shared data contracts. Stress the importance of separation of concerns, modularization, and consistency in code organization for maintainability.
Sample Answer: "For a large-scale TypeScript application, I would structure the codebase into well-defined modules, each encapsulating its own functionality. I would organize components into folders like /src/components, /src/services, and /src/utils, keeping logical boundaries clear. Additionally, I would define interfaces in a /types directory to ensure a consistent contract across modules. Each module would manage its dependencies and exports to prevent circular references, thereby optimizing maintainability and readability."
What to Look For: Candidates should convey their understanding of best practices in code organization specific to TypeScript. Strong responses will address the importance of clear structure and module separations while demonstrating the thought process behind their approach.
How to Answer: Acknowledge that while TypeScript adds a compilation step that can increase build time, its benefits on code maintainability and safety often outweigh these costs. Discuss ways to optimize performance, including incremental compilation and minimizing type definitions.
Sample Answer: "Using TypeScript can slightly impact performance due to the additional compilation step required to convert TypeScript into JavaScript. However, this trade-off is typically worth it for the benefits gained in maintainability and reduced errors. To optimize performance, I often utilize incremental compilation, which significantly reduces build times by recompiling only changed files. Additionally, maintaining clean type definitions and avoiding overly complex types helps in keeping both the development and runtime performance in check."
What to Look For: Candidates should balance acknowledging the potential downsides of TypeScript with a strong appreciation of its advantages. Watch for references to specific strategies for optimization and an understanding of the practical impacts of using TypeScript in real-world projects.
How to Answer: Explain that strict mode in TypeScript enforces stricter type checks and helps catch potential errors earlier in the development process. Discuss specific strict flags and their impacts, such as noImplicitAny and strictNullChecks.
Sample Answer: "Strict mode in TypeScript is invaluable as it enforces stricter type-checking rules across the codebase. Enabling noImplicitAny, for example, requires all variables to have a defined type, preventing TypeScript from inferring 'any' types, which can lead to runtime issues. Similarly, strictNullChecks ensures that null or undefined values do not flow through your code without explicit handling, drastically reducing the chances of null reference errors. By embracing strict mode, I can catch potential issues early, leading to more robust code production."
What to Look For: Look for candidates who understand the impact of strict checks on code safety and quality. Strong responses will include specific examples of how strict modes have benefited their projects.
How to Answer: Define type guards and how they enable developers to work with union types and improve type safety during runtime. Describe how they can be implemented through functions or using typeof or instanceof checks.
Sample Answer: "Type guards are a powerful feature in TypeScript that allows us to narrow down types at runtime, especially when dealing with union types. For instance, I might create a function function isString(value: any): value is string { return typeof value === 'string'; } which checks if a value is a string. By using this function, I can safely handle different types of input without TypeScript complaining about potential type issues, ensuring type safety and clarity in handling varied data types in a controlled manner."
What to Look For: Candidates should show a clear understanding of how type guards function within the TypeScript type system. Look for the ability to articulate their utility in achieving more precise type handling in practical scenarios.
How to Answer: Discuss strategies for defining types that match the expected structure of API responses. Highlight the benefit of using interfaces and types to ensure that application logic adheres to expected data shapes and prevents errors.
Sample Answer: "To ensure type safety when working with data from an API, I would define TypeScript interfaces that mirror the structure of the expected response. For instance, if an API for user data returns { id: number, name: string }, I would create an interface like interface User { id: number; name: string; }. Upon fetching the data, I can cast the response to this interface using const user: User = await response.json();. This approach helps me catch discrepancies between the expected and actual data structures early in development."
What to Look For: Look for candidates to express understanding of ensuring type safety through interfaces. Strong candidates will articulate a methodical approach to interfacing with APIs while focusing on preventing potential data-related bugs within their applications.
How to Answer: Explain that type inference allows TypeScript to automatically detect and assign types without explicit annotations. Discuss how it contributes to a smoother developer experience by reducing the verbosity of code while still retaining types.
Sample Answer: "Type inference in TypeScript is a powerful feature that allows the compiler to automatically infer types based on the values assigned to variables. For example, if I declare let age = 30;, TypeScript automatically infers that age is a number. This means I don't always have to explicitly state types, making my code cleaner and more concise while still benefiting from type safety. However, for more complex structures, I still ensure to define types to maintain clarity and prevent unintended behaviors."
What to Look For: Candidates should clearly understand type inference and its functionality in TypeScript. Strong candidates will provide examples demonstrating how inference has benefitted their coding practices, highlighting a balance between conciseness and clarity.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
TypeScript has gained significant traction in the software development industry, not just as an alternative to JavaScript but as a tool that provides additional functionality and robustness. Its adoption spans across various development areas, from frontend to backend, making it a versatile choice for modern applications. Let’s explore how TypeScript enhances development practices and why it has become a preferred language for many developers and organizations.
TypeScript's influence in both frontend and backend development is profound, offering benefits that improve code quality, maintainability, and developer productivity.
TypeScript's role in frontend development is particularly notable when working with popular frameworks and libraries. Here’s how TypeScript enhances frontend development:
On the backend, TypeScript’s benefits extend to server-side development, particularly with Node.js and various backend frameworks:
Switching from JavaScript to TypeScript offers several tangible benefits that can significantly enhance your development workflow and code quality. Here’s why TypeScript stands out:
TypeScript’s versatility makes it applicable to a wide range of scenarios in the industry. Here are some common use cases where TypeScript shines:
Overall, TypeScript’s ability to improve code quality, enhance development efficiency, and manage complexity makes it a valuable tool for developers across various domains and industries. Whether you are developing a frontend application, a backend service, or working with open source projects, understanding and utilizing TypeScript can provide significant advantages.
Mastering TypeScript requires a deep understanding of its core concepts, which provide the foundation for writing robust and maintainable code. Let’s explore these fundamental and advanced features, along with how to handle errors and debug effectively in TypeScript.
Understanding TypeScript’s fundamental features is crucial for writing clean, type-safe code. These core concepts enable you to leverage TypeScript’s full potential.
TypeScript introduces a static type system to JavaScript, allowing you to define types for variables, function parameters, and return values. This helps in catching errors during development rather than at runtime. Here are some basic types you should know:
number
, string
, boolean
, null
, undefined
, and symbol
. For example:let age: number = 25;
let name: string = "Alice";
let isActive: boolean = true;
let scores: number[] = [85, 90, 92];
let user: [string, number] = ["Alice", 25];
enum Direction {
Up,
Down,
Left,
Right
}
let move: Direction = Direction.Up;
Interfaces in TypeScript are used to define the shape of objects. They can include properties and methods and can be extended or implemented by classes. Here’s a basic example:
interface Person {
name: string;
age: number;
greet(): void;
}
const person: Person = {
name: "John",
age: 30,
greet() {
console.log(`Hello, my name is ${this.name}`);
}
};
Interfaces are also useful for defining complex data structures and ensuring that classes or objects adhere to a specific contract.
TypeScript enhances JavaScript’s class-based object-oriented programming by adding features such as access modifiers (public
, private
, protected
) and class decorators. Here’s an example of a TypeScript class:
class Animal {
private name: string;
constructor(name: string) {
this.name = name;
}
public makeSound(): void {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
public bark(): void {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog("Rex");
dog.makeSound(); // Rex makes a sound.
dog.bark(); // Rex barks.
This class-based approach helps in creating well-structured and reusable code.
To fully leverage TypeScript, you need to understand its advanced features. These concepts provide flexibility and power in your coding practices.
Generics enable you to write flexible, reusable functions and classes by allowing them to operate on types specified at runtime. They provide a way to capture the type of input and output, enhancing code reusability. Here’s a simple example:
function identity<T>(arg: T): T {
return arg;
}
let stringResult = identity<string>("Hello");
let numberResult = identity<number>(123);
Generics can also be used with classes:
class Box<T> {
private content: T;
constructor(content: T) {
this.content = content;
}
public getContent(): T {
return this.content;
}
}
let stringBox = new Box<string>("Hello");
let numberBox = new Box<number>(123);
Generics are particularly useful in libraries and frameworks, where you want to ensure type safety while allowing for diverse data types.
TypeScript can infer types based on the values you assign to variables or function return types. This reduces the need for explicit type annotations while still providing type safety. Here’s an example:
let message = "Hello, TypeScript"; // TypeScript infers 'message' as string
function multiply(x: number, y: number) {
return x * y; // TypeScript infers the return type as number
}
Type inference makes your code cleaner and easier to maintain without sacrificing type safety.
Union types allow a variable to hold values of multiple types. This is useful when you want to accept different types of input in a function or a variable. Here’s how you can use union types:
function formatValue(value: string | number): string {
if (typeof value === "string") {
return value.toUpperCase();
}
return value.toFixed(2);
}
let formattedString = formatValue("hello"); // Output: "HELLO"
let formattedNumber = formatValue(123.456); // Output: "123.46"
Union types provide flexibility while maintaining type safety by narrowing down the possible types at runtime.
Effective error handling and debugging are crucial for maintaining the stability of your TypeScript applications. TypeScript provides several tools and techniques to help with these aspects.
TypeScript’s type system helps prevent many runtime errors by enforcing type constraints during compilation. However, you should still handle runtime errors gracefully. Use try-catch
blocks to manage exceptions:
function safeDivide(a: number, b: number): number | null {
try {
if (b === 0) {
throw new Error("Division by zero");
}
return a / b;
} catch (error) {
console.error(error);
return null;
}
}
This example demonstrates how to handle errors that might occur during execution, ensuring your application can manage unexpected situations gracefully.
Debugging TypeScript code involves using the tools provided by TypeScript and modern development environments:
tsconfig.json
file to include options such as "strict": true
for rigorous type checking.By mastering these fundamental and advanced TypeScript concepts, and employing effective error handling and debugging strategies, you will be well-equipped to write reliable and maintainable TypeScript code. Understanding and applying these principles will enhance your development skills and contribute to the success of your projects.
When preparing for a TypeScript interview, it’s essential to focus on a range of topics that cover both basic syntax and more advanced programming constructs. Being well-versed in these areas will not only help you answer questions effectively but also demonstrate your proficiency in TypeScript.
Understanding TypeScript syntax and programming constructs is foundational for any TypeScript interview. This knowledge encompasses the core elements of the language that you'll frequently use in both everyday coding and interview scenarios.
Variable Declarations and Types
TypeScript allows you to declare variables with specific types, which enhances code clarity and safety. Here’s how you define variables with types:
let isActive: boolean = true;
let username: string = "Alice";
let age: number = 30;
let scores: number[] = [95, 85, 76];
let user: [string, number] = ["Bob", 28];
Functions in TypeScript can be typed to ensure that parameters and return values conform to expected types:
function greet(name: string): string {
return `Hello, ${name}`;
}
function buildGreeting(name: string, greeting: string = "Hi"): string {
return `${greeting}, ${name}`;
}
function sum(...numbers: number[]): number {
return numbers.reduce((acc, num) => acc + num, 0);
}
TypeScript provides the same control flow constructs as JavaScript but with added type safety:
let status: string = "success";
if (status === "success") {
console.log("Operation was successful.");
} else {
console.log("Operation failed.");
}
for
, while
, and for...of
:for (let i = 0; i < 5; i++) {
console.log(i);
}
Generics in TypeScript provide a way to create reusable components and functions that can operate on a variety of types. They allow you to write more flexible and type-safe code.
Generics enable functions and classes to work with different types while maintaining type safety. Here’s a basic example of a generic function:
function identity<T>(arg: T): T {
return arg;
}
let stringResult = identity<string>("Hello, world!");
let numberResult = identity<number>(123);
In this example, T
represents a generic type parameter, which is specified when the function is called.
Generics can also be used with classes to create flexible data structures:
class Box<T> {
private content: T;
constructor(content: T) {
this.content = content;
}
public getContent(): T {
return this.content;
}
}
let stringBox = new Box<string>("Hello");
let numberBox = new Box<number>(42);
Here, Box
is a generic class that can hold any type of content, enhancing its reusability.
Sometimes, you need to restrict the types that can be used with generics. Generic constraints allow you to specify that a type must meet certain criteria:
interface Lengthwise {
length: number;
}
function logLength<T extends Lengthwise>(item: T): void {
console.log(item.length);
}
logLength({ length: 10, value: "example" }); // Works
logLength("Hello"); // Works because string has a length property
In this example, T
is constrained to types that have a length
property, ensuring that logLength
can only be called with arguments that meet this criterion.
Writing maintainable and scalable TypeScript code involves adhering to best practices for design and architecture. These practices help ensure that your code is clean, understandable, and easy to manage.
Organize your code into modules and use TypeScript’s module system to keep your codebase manageable. Use import
and export
to share functionality between files:
// user.ts
export interface User {
name: string;
age: number;
}
// userService.ts
import { User } from "./user";
export function createUser(name: string, age: number): User {
return { name, age };
}
Leverage TypeScript’s type system to define clear contracts for your functions and objects. This makes your code more predictable and less prone to errors:
interface Product {
id: number;
name: string;
price: number;
}
function printProduct(product: Product): void {
console.log(`Product: ${product.name}, Price: $${product.price}`);
}
Separate concerns by organizing your code into distinct layers or modules, such as data access, business logic, and presentation. This helps maintain a clear separation of responsibilities:
Maintain consistency in your codebase by adhering to a style guide and using linters. This helps improve readability and reduces errors:
Testing is crucial for ensuring the reliability of your TypeScript code. Write unit tests to cover individual components and functions:
import { createUser } from "./userService";
test("createUser should return a user with the given name and age", () => {
const user = createUser("Alice", 30);
expect(user.name).toBe("Alice");
expect(user.age).toBe(30);
});
Utilize testing frameworks like Jest or Mocha to automate and manage your tests effectively.
By mastering these essential TypeScript syntax and constructs, understanding generics, and applying best practices for code design, you will be well-prepared for TypeScript interviews and equipped to write high-quality, maintainable code. These skills are fundamental in demonstrating your expertise and understanding of TypeScript in both technical assessments and real-world applications.
Preparing for a TypeScript interview involves a multifaceted approach. You need to refresh your knowledge of core concepts, practice coding problems, and understand the types of questions that might be asked. Here are some key steps to ensure you're well-prepared:
interface
and type
, using generics effectively, and handling type errors.
Assessing TypeScript proficiency during interviews involves evaluating both technical skills and practical experience. To ensure a thorough evaluation, consider these key areas:
When working with TypeScript, both newcomers and experienced developers can encounter pitfalls that affect code quality and maintainability. Here’s a list of common mistakes and tips on how to avoid them:
any
excessively, as it defeats the purpose of TypeScript’s type safety. Instead, use specific types or generics to maintain type safety and clarity.By addressing these common mistakes and focusing on effective interview preparation and evaluation techniques, you’ll enhance your TypeScript skills and improve your ability to assess and work with TypeScript effectively.
For experienced candidates, TypeScript proficiency goes beyond the basics. It involves deep diving into real-world scenarios, understanding its use in complex applications and frameworks, and preparing for advanced interview questions. This section covers these advanced topics to help you showcase your expertise and tackle higher-level challenges in TypeScript.
Advanced TypeScript skills involve applying the language to solve complex problems and optimize real-world applications. Here are some scenarios and techniques that experienced candidates should be familiar with:
Type guards are functions that help TypeScript narrow down the type of a variable within a conditional block. Custom type guards can enhance type safety in complex scenarios:
function isString(value: any): value is string {
return typeof value === "string";
}
function printValue(value: string | number): void {
if (isString(value)) {
console.log(value.toUpperCase());
} else {
console.log(value.toFixed(2));
}
}
Custom type guards provide more precise type information and help ensure that your code handles different data types correctly.
TypeScript allows for sophisticated type manipulations using utility types and conditional types. For example:
type IsString<T> = T extends string ? "Yes" : "No";
type Test1 = IsString<string>; // "Yes"
type Test2 = IsString<number>; // "No"
type ReadOnly<T> = {
readonly [K in keyof T]: T[K];
};
interface User {
name: string;
age: number;
}
type ReadOnlyUser = ReadOnly<User>;
TypeScript supports decorators, which are special kinds of declarations that can be attached to classes, methods, or properties. They provide a way to modify or extend functionality:
function Log(target: any, propertyKey: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
descriptor.value = function (...args: any[]) {
console.log(`Arguments: ${args}`);
return originalMethod.apply(this, args);
};
}
class Calculator {
@Log
add(a: number, b: number): number {
return a + b;
}
}
Decorators are used in frameworks like Angular for dependency injection and other features.
TypeScript’s application in complex systems and frameworks highlights its strengths in managing large codebases and enhancing development efficiency.
React, a popular frontend library, integrates seamlessly with TypeScript. It provides static typing for components, props, and state, enhancing code quality:
interface Props {
message: string;
}
const Greeting: React.FC<Props> = ({ message }) => {
return <h1>{message}</h1>;
};
TypeScript helps catch errors related to props and state early, improving component reliability.
In server-side development with Node.js, TypeScript can improve the management of asynchronous operations and API interactions:
import express, { Request, Response } from "express";
const app = express();
app.get("/", (req: Request, res: Response) => {
res.send("Hello, world!");
});
async function fetchData(url: string): Promise<string> {
const response = await fetch(url);
const data = await response.json();
return data.message;
}
For large-scale applications, TypeScript helps in managing complexity through:
Senior TypeScript roles often require a deep understanding of the language and its application in complex scenarios. Here are some advanced interview questions that can help assess a candidate’s expertise:
tsconfig.json
that improve type checking, module resolution, and error reporting.By mastering these advanced topics and preparing for senior-level interview questions, you can demonstrate a high level of expertise in TypeScript and its application in complex scenarios. This preparation will help you excel in interviews and showcase your ability to handle sophisticated TypeScript challenges.
Mastering TypeScript interview questions involves more than just understanding the language’s syntax; it requires a deep comprehension of how TypeScript enhances development practices and how to apply its features effectively in real-world scenarios. By focusing on core concepts, advanced features, and common interview topics, you can showcase your ability to write clean, maintainable code and solve complex problems with TypeScript. Whether you’re preparing for an interview or evaluating candidates, understanding TypeScript’s role in modern development and practicing relevant questions will help you stand out and demonstrate your proficiency.
Effective preparation not only involves reviewing technical details but also practicing problem-solving and coding exercises. Familiarizing yourself with common pitfalls and best practices ensures that you are ready to tackle any challenge that comes your way. For employers, using structured interview techniques and clear criteria will help in assessing candidates' skills accurately. By applying the insights from this guide, you can approach TypeScript interviews with confidence, ensuring that you are well-prepared to succeed or find the right talent for your team.