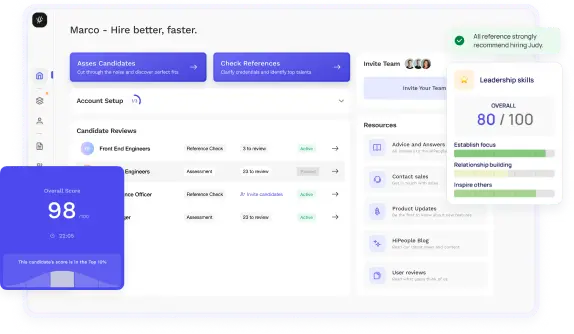
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to demonstrate your Python Object-Oriented Programming (OOP) expertise in interviews? To help you succeed, we've compiled a guide that dives deep into Python OOPS interview questions and covers key concepts, best practices, and real-world scenarios.
Whether you're a seasoned Python developer or just starting your coding career, this guide will equip you with the knowledge and confidence to tackle OOP-related questions and showcase your OOP skills effectively in interviews.
Python Object-Oriented Programming (OOP) interview questions are commonly asked by employers and technical interviewers to assess a candidate's knowledge and proficiency in using OOP principles and concepts in Python. These questions aim to evaluate your understanding of classes, objects, inheritance, encapsulation, polymorphism, design patterns, and best practices related to OOP in Python.
Interview questions related to Python OOP can vary in complexity, from basic questions that test your understanding of OOP fundamentals to more advanced questions that require you to solve real-world problems using OOP techniques.
In a Python OOP interview, you can expect questions that cover a range of topics and concepts. Here are the key elements that are often assessed:
Python OOP interviews are significant for both job seekers and employers:
Python OOP interviews play a crucial role in assessing and validating a candidate's proficiency in object-oriented programming, ensuring that the right individuals are selected for roles that require strong Python OOP skills.
We'll explore the fundamental Python OOP concepts and principles in more detail. These principles are the building blocks of object-oriented programming and form the foundation for writing efficient and maintainable code.
Classes in Python are user-defined data types that allow you to create objects. An object is an instance of a class, and it encapsulates both data (attributes) and behavior (methods). Classes are defined using the class keyword.
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return f"{self.name} barks loudly!"
You can create instances of the Dog class, each with its own set of attributes and methods:
my_dog = Dog("Buddy", "Golden Retriever")
print(my_dog.name) # Output: Buddy
print(my_dog.bark()) # Output: Buddy barks loudly!
Inheritance is a powerful concept in OOP that allows you to create a new class (the subclass or derived class) based on an existing class (the superclass or base class). The derived class inherits attributes and methods from the base class, promoting code reuse and hierarchical organization.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass # Abstract method, to be implemented by subclasses
class Dog(Animal):
def speak(self):
return f"{self.name} barks!"
In this example, the Dog class is derived from the Animal class, inheriting its name attribute and speak() method.
Encapsulation is the concept of bundling data (attributes) and the methods (functions) that operate on that data into a single unit called a class. It enforces the idea that the internal details of a class should be hidden from the outside world, allowing controlled access through defined interfaces.
class BankAccount:
def __init__(self, account_number, balance):
self._account_number = account_number # Protected attribute
self.__balance = balance # Private attribute
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
Polymorphism is the ability of different classes to be treated as instances of a common base class. It enables you to write code that can work with objects of various classes without knowing their specific types.
class Shape:
def area(self):
pass # Abstract method, to be implemented by subclasses
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
Abstraction is the process of simplifying complex systems by modeling classes based on real-world entities and exposing only the essential details. It involves defining a clear and concise interface while hiding the underlying complexity.
Abstraction is achieved by creating classes that provide a well-defined set of methods and attributes for interaction, abstracting away the intricate implementation details.
Now, we'll take a deeper dive into key Object-Oriented Programming (OOP) features in Python. These features are essential for writing efficient, extensible, and maintainable code.
A constructor in Python is a special method called __init__() that is automatically invoked when an object of a class is created. Constructors initialize the attributes and perform any necessary setup for objects.
class MyClass:
def __init__(self, param1, param2):
self.param1 = param1
self.param2 = param2
# Creating an instance of MyClass
obj = MyClass("Hello", 42)
In this example, the __init__() constructor initializes the param1 and param2 attributes when an instance of MyClass is created.
While Python automatically manages memory, you can use a destructor method called __del__() to perform cleanup tasks before an object is destroyed. This is less commonly used than constructors.
class MyClass:
def __init__(self):
print("Constructor called")
def __del__(self):
print("Destructor called")
obj1 = MyClass() # Constructor called
del obj1 # Destructor called
The __del__() method is called when an object is about to be removed from memory, allowing you to release resources or perform cleanup operations.
Method overriding is a feature in Python OOP that allows a subclass to provide a specific implementation of a method that is already defined in its superclass. This enables the subclass to customize or extend the behavior inherited from the superclass.
class Animal:
def speak(self):
pass # Abstract method, to be implemented by subclasses
class Dog(Animal):
def speak(self):
return "Woof!"
Method overriding is a powerful mechanism for creating specialized behavior in subclasses while maintaining a common interface.
Class variables are shared among all instances of a class. They are defined within the class but outside any method. Class variables are associated with the class itself, not with instances of the class.
class MyClass:
class_var = 0 # Class variable
def __init__(self, instance_var):
self.instance_var = instance_var # Instance variable
In this example, class_var is a class variable that can be accessed using the class name MyClass.class_var. It is shared among all instances of MyClass.
Instance variables are unique to each instance of a class. They are defined within the class's methods and are specific to individual objects.
class MyClass:
class_var = 0 # Class variable
def __init__(self, instance_var):
self.instance_var = instance_var # Instance variable
Here, instance_var is an instance variable that is specific to each object created from the MyClass class. Each instance of MyClass can have a different value for instance_var.
Understanding the distinction between class variables and instance variables is essential for effective OOP design.
Access modifiers in Python are conventions used to control the visibility of class members (attributes and methods). While Python does not enforce strict access control like some other languages, it provides naming conventions to indicate the visibility of class members:
class MyClass:
public_var = 42 # Public member
_protected_var = 21 # Protected member
__private_var = 7 # Private member
It's important to note that these conventions are not enforced by the Python interpreter but serve as a guideline for developers.
Decorators in Python are functions that modify the behavior of other functions or methods. They are often used in OOP to add functionality to methods or to control access to them.
def validate_positive(func):
def wrapper(instance, value):
if value < 0:
raise ValueError("Value must be positive")
return func(instance, value)
return wrapper
class BankAccount:
def __init__(self, balance):
self.balance = balance
@validate_positive
def deposit(self, amount):
self.balance += amount
In this example, the @validate_positive decorator ensures that the deposit() method of the BankAccount class only accepts positive values. Decorators are a powerful tool for extending and enhancing the functionality of methods in a clean and modular way.
These key OOP features provide you with the tools and techniques needed to create well-structured, maintainable, and extensible Python code.
How to Answer: Start by explaining that the four fundamental principles of OOP are encapsulation, inheritance, polymorphism, and abstraction. Provide a brief definition and example for each principle to demonstrate your understanding.
Sample Answer:"The four fundamental principles of OOP are:
What to Look For: Look for a clear and concise explanation of each OOP principle with relevant examples. Candidates should demonstrate their understanding of these core concepts and how they apply them in Python.
How to Answer: Explain that a class is a blueprint or template for creating objects, while an object is an instance of a class. Describe how classes define attributes and methods, while objects represent specific instances with their unique attribute values.
Sample Answer:"In Python, a class is a blueprint or a template for creating objects. A class defines the attributes (data) and methods (functions) that its objects will have. Think of a class as a 'recipe' for creating objects of a specific type.
An object, on the other hand, is a specific instance of a class. It represents a real-world entity or concept and has its unique attribute values. You can create multiple objects from the same class, each with its own set of attribute values.
For example, consider a 'Person' class. The class defines attributes like 'name' and 'age' and methods like 'eat' and 'sleep.' When we create objects from this class, such as 'person1' and 'person2,' they will have their individual 'name' and 'age' values."
What to Look For: Ensure the candidate can clearly distinguish between classes and objects, explaining their roles and how they relate in Python.
How to Answer: Describe encapsulation as the concept of bundling data and methods within a class while controlling access to them. Explain that encapsulation is achieved through access modifiers like private, protected, and public in Python.
Sample Answer:"Encapsulation in Python is the practice of bundling data (attributes) and methods (functions) that operate on that data into a single unit, called a class. It restricts access to the internal state of objects, promoting data integrity and security.
In Python, encapsulation is implemented using access modifiers:
By using access modifiers, Python allows developers to control the visibility of class members, ensuring that sensitive data and methods are not accessible directly from outside the class."
What to Look For: Assess the candidate's understanding of encapsulation and their ability to explain how it is implemented in Python using access modifiers.
How to Answer: Define inheritance as the mechanism that allows a class to inherit properties and methods from another class. Explain its importance in promoting code reuse and structuring classes hierarchically.
Sample Answer:Inheritance is a fundamental concept in Object-Oriented Programming (OOP) that allows a class (subclass or derived class) to inherit properties and methods from another class (base class or parent class). It is important in OOP for the following reasons:
What to Look For: Look for a comprehensive explanation of inheritance and its significance in OOP, including benefits like code reuse, hierarchical structure, polymorphism, and modularity.
How to Answer: Explain that method overriding allows a subclass to provide a specific implementation of a method inherited from its superclass. Describe the use of the super() function and the @abstractmethod decorator (if applicable).
Sample Answer:Method overriding in Python allows a subclass to provide a specific implementation of a method that is already defined in its superclass. To override a method, follow these steps:
Here's an example:
class Animal:
def speak(self):
print("Animal speaks")
class Dog(Animal):
def speak(self):
super().speak() # Call the superclass method
print("Dog barks")
# Create instances
animal = Animal()
dog = Dog()
# Method calls
animal.speak() # Output: "Animal speaks"
dog.speak() # Output: "Animal speaks" followed by "Dog barks"
In this example, the Dog class overrides the speak() method inherited from the Animal class and extends its behavior by adding "Dog barks" to the output."
What to Look For: Ensure the candidate understands the concept of method overriding, can provide a clear example, and is aware of the use of super() to call the superclass's method.
How to Answer: Define abstraction as the process of simplifying complex reality by modeling classes based on essential properties and behaviors. Explain how abstraction relates to polymorphism by defining abstract classes and methods.
Sample Answer:Abstraction is the process of simplifying complex reality by modeling classes based on essential properties and behaviors while hiding unnecessary details. It involves creating abstract classes with some methods declared but not implemented. Abstraction is related to polymorphism in the following way:
Here's a simplified example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
class Rectangle(Shape):
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.length * self.width
In this example, the Shape class is abstract, and it defines an abstract method area(). Both Circle and Rectangle subclasses implement the area() method in their unique ways, achieving polymorphism."
What to Look For: Assess the candidate's understanding of abstraction and its connection to polymorphism. Ensure they can explain the concept and provide an example involving abstract classes and methods.
How to Answer: Define polymorphism as the ability of objects of different classes to be treated as objects of a common superclass. Explain its usefulness in simplifying code and promoting flexibility.
Sample Answer:Polymorphism in Python refers to the ability of objects of different classes to be treated as objects of a common superclass. It is useful for the following reasons:
For example, consider a scenario where you have different types of shapes (e.g., circles, rectangles) that all have a common method calculate_area(). Polymorphism enables you to write code that calculates the area of any shape without knowing its specific type."
What to Look For: Look for a clear explanation of polymorphism, its benefits in terms of code flexibility and simplification, and the mention of dynamic binding.
How to Answer: Describe encapsulation as the bundling of data and methods within a class while controlling access using access modifiers. Provide examples of access modifiers such as private, protected, and public.
Sample Answer:Encapsulation in Python is the concept of bundling data (attributes) and methods (functions) that operate on that data into a single unit, called a class. It helps in controlling access to the internal state of objects and ensures data integrity and security. Encapsulation is implemented using access modifiers:
Here's an example to illustrate encapsulation:
class Student:
def __init__(self, name, roll_number):
self.__name = name # Private attribute
self._roll_number = roll_number # Protected attribute
def display(self):
print(f"Name: {self.__name}, Roll Number: {self._roll_number}")
# Creating an instance of Student
student = Student("Alice", 101)
# Accessing attributes (not recommended, but possible)
print(student._roll_number) # Protected attribute (valid but not recommended)
print(student.__name) # Error: 'Student' object has no attribute '__name'
# Accessing attributes using public method
student.display() # Output: "Name: Alice, Roll Number: 101"
In this example, the name attribute is private, and the roll_number attribute is protected. Access to these attributes is controlled through the display() method."
What to Look For: Assess the candidate's understanding of encapsulation and their ability to explain how it is implemented using access modifiers. Ensure they understand the importance of data privacy.
How to Answer: Explain that private attributes enhance data encapsulation and security. Mention benefits like preventing accidental modification and enabling controlled access through methods.
Sample Answer:Using private attributes in Python classes offers several advantages:
What to Look For: Look for candidates to explain the advantages of private attributes in terms of data encapsulation, prevention of accidental modification, controlled access, code maintainability, and security.
How to Answer: Explain that Python does not support traditional method overloading based on the number or type of arguments. Describe how method overloading can be achieved using default arguments or variable-length argument lists.
Sample Answer:Python does not support traditional method overloading, where you can define multiple methods with the same name but different parameter lists, as you might do in some other programming languages. However, method overloading can be achieved in Python using default arguments or variable-length argument lists (e.g., *args and **kwargs).
Here's an example using default arguments:
class Calculator:
def add(self, a, b=0):
return a + b
calc = Calculator()
result1 = calc.add(5) # Calls add(a, b=0), result1 = 5
result2 = calc.add(2, 3) # Calls add(a, b), result2 = 5
In this example, the add() method can accept one or two arguments. If only one argument is provided, it defaults to 0 for the second argument. This achieves method overloading.
What to Look For: Assess the candidate's understanding of method overloading in Python, their ability to explain how it can be achieved, and their provision of a clear example.
How to Answer: Explain that the super() function is used to call a method from the parent class (superclass) within a subclass. Describe how it is commonly used to initialize attributes in the subclass's constructor.
Sample Answer:The super() function in Python is used to call a method from the parent class (superclass) within a subclass. Its primary purpose is to access and invoke methods or attributes defined in the superclass. It is commonly used in the constructor of a subclass to initialize attributes inherited from the superclass.
For example, consider a class hierarchy with a base class Vehicle and a subclass Car:
class Vehicle:
def __init__(self, brand):
self.brand = brand
class Car(Vehicle):
def __init__(self, brand, model):
super().__init__(brand) # Call the superclass constructor
self.model = model
# Creating an instance of Car
my_car = Car("Toyota", "Camry")
In this example, the super().__init__(brand) line within the Car class constructor calls the constructor of the Vehicle superclass, allowing the Car object to initialize the brand attribute inherited from Vehicle.
What to Look For: Ensure the candidate understands the purpose of the super() function, especially in the context of initializing attributes in a subclass's constructor.
How to Answer: Explain that composition is a design principle where a class contains instances of other classes as attributes, while inheritance is a mechanism for a class to inherit properties and methods from another class. Describe the advantages and disadvantages of each approach.
Sample Answer:Composition and inheritance are two different approaches in object-oriented programming:
Composition:
Inheritance:
The choice between composition and inheritance depends on the specific requirements of a design. Composition is often preferred when flexibility and modularity are key considerations, while inheritance is suitable for situations where code reuse and an "is-a" relationship are more appropriate.
What to Look For: Assess the candidate's understanding of the differences between composition and inheritance and their ability to explain the advantages and disadvantages of each approach.
How to Answer: Define a constructor as a special method that initializes the attributes of an object when it is created. Explain its importance in ensuring that objects start with valid initial states.
Sample Answer:A constructor in Python is a special method used to initialize the attributes of an object when it is created. The constructor method is named __init__() and is automatically called when an object is instantiated from a class.
The importance of constructors in object-oriented programming includes:
Here's an example of a constructor in a Python class:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
# Creating an instance of Person with the constructor
person = Person("Alice", 30)
In this example, the __init__() constructor initializes the name and age attributes of the Person object.
What to Look For: Ensure the candidate can define a constructor, explain its importance, and provide an example of its usage.
How to Answer: Describe method chaining as a technique where multiple methods are called on an object in a single line of code, with each method returning the modified object. Explain its usefulness in writing concise and readable code.
Sample Answer:Method chaining in Python is a programming technique where multiple methods are called on an object in a single line of code, and each method returns the modified object. This allows you to chain together a sequence of method calls on an object, making code more concise and readable.
Method chaining is useful for several reasons:
Here's an example of method chaining in Python using a fictional StringBuilder class:
class StringBuilder:
def __init__(self):
self.value = ""
def append(self, text):
self.value += text
return self # Return the modified object
# Method chaining example
result = StringBuilder().append("Hello, ").append("world!").append(" How are you?").value
print(result) # Output: "Hello, world! How are you?"
In this example, each append() method returns the modified StringBuilder object, allowing multiple append() calls to be chained together in a single line.
What to Look For: Check if the candidate can explain what method chaining is, its benefits in terms of readability and conciseness, and provide a relevant example.
How to Answer: Describe the Global Interpreter Lock (GIL) as a mutex that allows only one thread to execute Python bytecode at a time in a multi-threaded Python program. Explain its impact on CPU-bound and I/O-bound tasks.
Sample Answer:The Global Interpreter Lock (GIL) in Python is a mutex (short for mutual exclusion) that allows only one thread to execute Python bytecode at a time, even in multi-threaded Python programs. The GIL is present in implementations of Python like CPython (the standard Python interpreter).
The impact of the GIL on multi-threading includes:
It's important to note that the GIL is specific to CPython and does not affect other implementations of Python, such as Jython or IronPython. Additionally, multi-processing (using multiple processes instead of threads) is a common approach to bypass the GIL and achieve true parallelism in CPU-bound tasks.
What to Look For: Assess the candidate's understanding of the Global Interpreter Lock (GIL), its impact on multi-threading, and their awareness of its relevance in CPU-bound and I/O-bound scenarios.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
We will delve into common design patterns used in Python Object-Oriented Programming (OOP). Design patterns are reusable solutions to common programming problems that help improve code organization, maintainability, and scalability. Understanding these patterns is valuable for designing robust and efficient software.
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to that instance. This can be useful when you need to control access to resources such as database connections, configuration settings, or a central manager.
Here's a Python implementation of the Singleton Pattern:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
By overriding the __new__() method, we ensure that only one instance of the Singleton class can be created.
The Factory Pattern is a creational design pattern that provides an interface for creating objects in a super class but allows subclasses to alter the type of objects that will be created. It promotes loose coupling between the client code and the objects it creates.
class ShapeFactory:
def create_shape(self, shape_type):
if shape_type == 'circle':
return Circle()
elif shape_type == 'rectangle':
return Rectangle()
else:
raise ValueError(f"Invalid shape type: {shape_type}")
The ShapeFactory class abstracts the creation of shapes, allowing clients to request shapes without needing to know the concrete implementation details.
The Decorator Pattern is a structural design pattern that allows you to add new behaviors or responsibilities to objects dynamically without altering their structure. Decorators are often used to extend the functionality of classes in a flexible and reusable way.
Here's an example of the Decorator Pattern in Python:
class Coffee:
def cost(self):
return 5
class MilkDecorator:
def __init__(self, coffee):
self._coffee = coffee
def cost(self):
return self._coffee.cost() + 2
In this example, Coffee is the base class, and MilkDecorator decorates a coffee object with the cost of adding milk. You can add more decorators like SugarDecorator, WhippedCreamDecorator, etc., to create various combinations.
The Observer Pattern is a behavioral design pattern that defines a one-to-many dependency between objects. When one object (the subject) changes state, all its dependents (observers) are notified and updated automatically.
Here's a simplified implementation of the Observer Pattern in Python:
class Subject:
def __init__(self):
self._observers = []
def attach(self, observer):
self._observers.append(observer)
def notify(self):
for observer in self._observers:
observer.update()
class Observer:
def update(self):
print("Observer has been notified.")
In this example, the Subject maintains a list of observers and notifies them when its state changes. Observers, represented by the Observer class, can be added to or removed from the subject as needed.
The Strategy Pattern is a behavioral design pattern that defines a family of algorithms, encapsulates each one, and makes them interchangeable. It allows clients to choose the appropriate algorithm at runtime, promoting flexibility and extensibility.
Here's an example of the Strategy Pattern in Python:
class PaymentStrategy:
def pay(self, amount):
pass
class CreditCardPayment(PaymentStrategy):
def pay(self, amount):
print(f"Paid ${amount} via credit card")
class PayPalPayment(PaymentStrategy):
def pay(self, amount):
print(f"Paid ${amount} via PayPal")
In this example, PaymentStrategy is the strategy interface, and CreditCardPayment and PayPalPayment are concrete strategies. Depending on the chosen payment method, you can select and use the appropriate strategy.
Design patterns like these are essential tools for designing robust and maintainable software. By incorporating these patterns into your Python OOP code, you can improve code structure, maintainability, and scalability while adhering to best practices in software design.
Let's explore practical examples of Python Object-Oriented Programming (OOP) concepts. We'll cover creating and using classes, implementing inheritance, working with encapsulation and abstraction, applying polymorphism, and showcasing real-world design pattern implementations.
Creating and using classes is fundamental to OOP. Classes allow you to model real-world entities and define their attributes and behaviors. Let's consider an example where we create a Person class:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f"Hello, my name is {self.name} and I am {self.age} years old."
# Creating instances of the Person class
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
# Using methods and accessing attributes
print(person1.greet()) # Output: Hello, my name is Alice and I am 30 years old.
print(person2.greet()) # Output: Hello, my name is Bob and I am 25 years old.
In this example, we create a Person class with attributes name and age, as well as a greet() method for introducing themselves.
Inheritance is a powerful concept that allows you to create new classes based on existing classes, promoting code reuse. Let's create a hierarchy of Shape classes:
class Shape:
def __init__(self, color):
self.color = color
def area(self):
pass # Abstract method, to be implemented by subclasses
class Circle(Shape):
def __init__(self, color, radius):
super().__init__(color)
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
class Rectangle(Shape):
def __init__(self, color, width, height):
super().__init__(color)
self.width = width
self.height = height
def area(self):
return self.width * self.height
Here, Shape is the base class, and Circle and Rectangle are derived classes. Each subclass inherits the color attribute and implements its own area() method.
Encapsulation and abstraction help in organizing and hiding the implementation details of a class. Let's consider a bank account example:
class BankAccount:
def __init__(self, account_number, balance):
self._account_number = account_number # Protected attribute
self.__balance = balance # Private attribute
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
In this example, we use _account_number as a protected attribute and __balance as a private attribute, demonstrating encapsulation. Abstraction comes into play by providing a clean interface for depositing and checking the balance, abstracting away the internal implementation.
Polymorphism allows objects of different classes to be treated as instances of a common base class. Let's see polymorphism in action with the speak() method:
class Animal:
def speak(self):
pass # Abstract method, to be implemented by subclasses
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
# Using polymorphism
def animal_sound(animal):
return animal.speak()
dog = Dog()
cat = Cat()
print(animal_sound(dog)) # Output: Woof!
print(animal_sound(cat)) # Output: Meow!
In this example, both Dog and Cat inherit from Animal, and they provide their own implementations of the speak() method. The animal_sound() function demonstrates polymorphism by accepting different animal objects.
Design patterns play a crucial role in structuring and optimizing code. Let's take a look at a practical implementation of the Singleton Pattern:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
This Singleton class ensures that only one instance of it can be created, no matter how many times you try to instantiate it.
These practical examples illustrate how you can apply various OOP concepts and design patterns in Python to create organized, reusable, and efficient code. Understanding and implementing these concepts is essential for becoming proficient in Python OOP development.
Finally, let's look at best practices for Python Object-Oriented Programming (OOP). Following these practices ensures that your OOP code is clean, maintainable, and efficient.
PEP 8 is the Python Enhancement Proposal that provides coding style guidelines for writing readable and consistent Python code. Adhering to PEP 8 is essential for writing clean and maintainable OOP code.
By adhering to PEP 8, your code will be more readable and easier to collaborate on with other developers.
Code reusability and maintainability are crucial aspects of OOP.
Unit testing is essential for verifying the correctness of your OOP code and ensuring that changes do not introduce regressions.
Good documentation and comments enhance the clarity and maintainability of your OOP code.
While writing OOP code, it's essential to consider performance optimizations when dealing with large-scale applications.
By following these best practices, you can ensure that your Python OOP code is not only clean and maintainable but also performs efficiently in production environments.
Mastering Python Object-Oriented Programming (OOP) concepts is essential for excelling in interviews and building robust software. With a solid understanding of classes, inheritance, encapsulation, polymorphism, and design patterns, you're well-prepared to answer OOP-related interview questions confidently. Remember to follow best practices, write clean code, and practice your problem-solving skills. Whether you're pursuing a career in Python development, data science, or automation, your OOP proficiency will be a valuable asset.
Furthermore, the importance of Python OOPS interviews cannot be overstated. For job seekers, these interviews provide opportunities to showcase their skills, gain a competitive edge, and secure rewarding positions in the tech industry. For employers, OOP interviews help identify candidates who can write maintainable, efficient code and contribute to the success of their organizations. By embracing Python OOP and preparing effectively for interviews, you're taking a significant step toward career advancement and success in the dynamic world of programming.